Before you jump into this, please note "3D Modeling is really time
consuming"!! It took almost two weeks for me to create the CPU Meter
background image! If you don't have enough time, I'd strongly recommend
you to stay out of the 3D modeling!!
Ok, now you are pretty sure to go one step forward:-)
There are many 3D modeling software, but most of them are pretty
expensive. If you have tons of money, buy what ever you want and take a
training course. But, fortunately, there is greate free 3D modeling
software, "Blender". I used "Blender" to make this application, but
"Blender" is designed as multi-platform software, and it has very unique
GUI and operations. If you know someone who is familiar with "Blender",
please do not hesitate to ask him how to use "Blender"! It's so unusual!
- Go to Blender.Org and install the latest Blender.
- Go to Blender Documentation and read the manual!
- Enjoy 3D modeling with Blender.
Now I'm assuming that you can create following 3D image with
Blender.
When you download "3DCPUMeter.blend"
and double click, following "Blender" will come up.
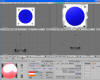
Now, press F12 key to render current frame. Then, following Render
image will be created.
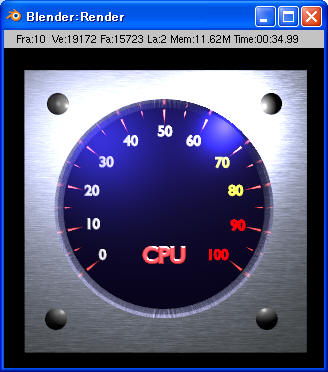
Press F3 key to save image as "3DCPU.png".
Please trim black background and save.
Now, finally you can get following background image!
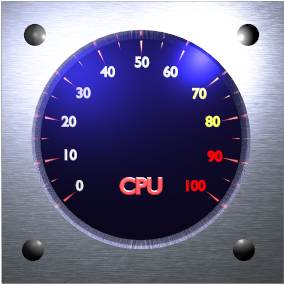