|
|
|
Windows.Forms.Form の基礎について説明します。 |
|
(1) VisualStudio.NET のヘルプ |
|
ダイアログのスタイルは、ダイアログのプロパティの "FormBorderStyle" で指定します(下図)。
None
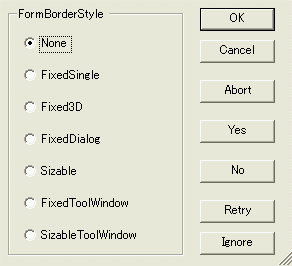
FixedSingle
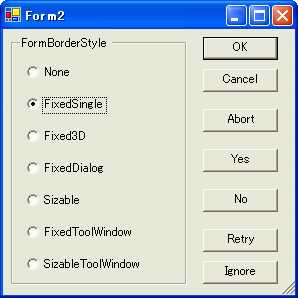
Fixed3D
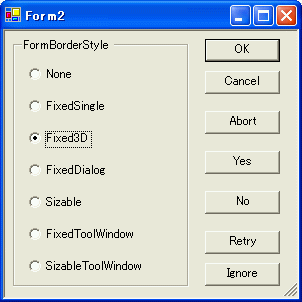
FixedDialog
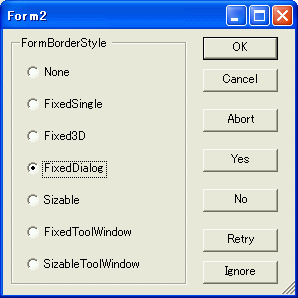
Sizable
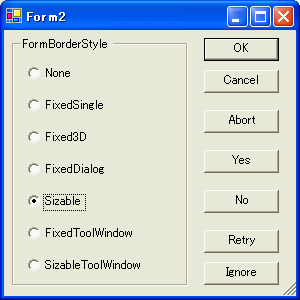
FixedToolWindow
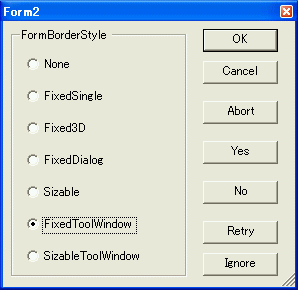
SizableToolWindow
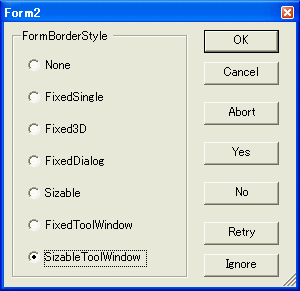
|
|
ダイアログには、ダイアログが終了するまで他のウィンドウの操作ができないモーダルダイアログと、MDIのようなモードレスダイアログがあります。
モード | 動作 |
モーダル ダイアログ | モーダルダイアログを表示している間は、常にモーダルダイアログが主で、モーダルダイアログを起動したもともとものメインウィンドウでのキーボードやマウスのクリックはできません。 |
モードレス ダイアログ |
これに対し、モードレスダイアログでは、メインウィンドウとモードレスダイアログの
どちらにでも、キーボードやマウスのクリックができます。 |
|
|
手順 | 作り方 |
1 | プロジェクトから Windows.Forms 新規プロジェクト (Dialogs1) を作成します。 これが親ウィンドウになります。 メインウィンドウは、デフォルトで Form1.cs になっているはずです。 |
2 |
ソリューションエクスプローラで、Dialog1 プロジェクトを右クリックし、追加→Windowsフォームの追加を選択します。
すると、新しい項目の追加ダイアログが表示されます。(下図)
ここで、テンプレートより、Windows フォームを選択し、Form2.cs を追加します。
これが、子ウィンドウになります。
すると、Windows.Forms.Form から継承した Form2 クラス (ファイルは Form2.cs) がプロジェクトに追加されます。
ここでは、この Form2 をモーダルダイアログで表示するようにします。
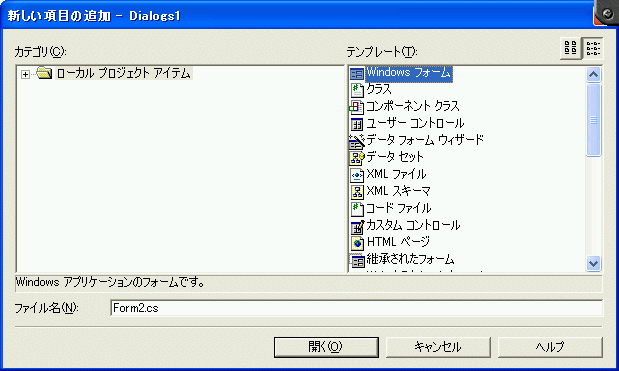 |
3 | メインのフォームウィンドウより、次のように ShowDialog()
で、モーダルダイアログ(Form2.cs) を起動します。 モーダルダイアログが閉じるまで、ShowDialog() からの制御は返りません。 ShowDialog() の返り値は、DialogResult です。この返り値に応じて適切な処理を実装します。 この DialogResult の返り値は、OK, Cance, Yes, No, Abort, Ignore, Retry, None
のおなじみの値が定義されています。
Form modalDialog = new Form2();
DialogResult result = modalDialog.ShowDialog();
switch(result)
{
case DialogResult.OK:
// 適当な処理
break;
case DialogResult.Cancel:
// 適当な処理
break;
case DialogResult.Yes:
// 適当な処理
break;
case DialogResult.No:
// 適当な処理
break;
case DialogResult.Abort:
// 適当な処理
break;
case DialogResult.Ignore:
// 適当な処理
break;
case DialogResult.Retry:
// 適当な処理
break;
case DialogResult.None:
// 適当な処理
break;
}
|
4 | モーダルダイアログに、OK, Cance, Yes, No, Abort, Ignore, Retry, None
ボタンを作ります。 まずは、Form2.cs のフォームデザイナーで、ツールバーより ボタンを7つ配置し、それぞれ ボタンのテキストに OK, Cancel
などとセットします。
次に、OKボタンのプロパティを下図のように開きます。"DialogResult"
というボタンの属性があるので、そのドロップダウンリストの中から、OK
を選びます。これだけで、このボタンを押しただけで、ダイアログが閉じて、ShowDialog()
の返り値として DialogResult.OK が返ります。
同様に、Cancel, Yes, No ....とセットしていきます。
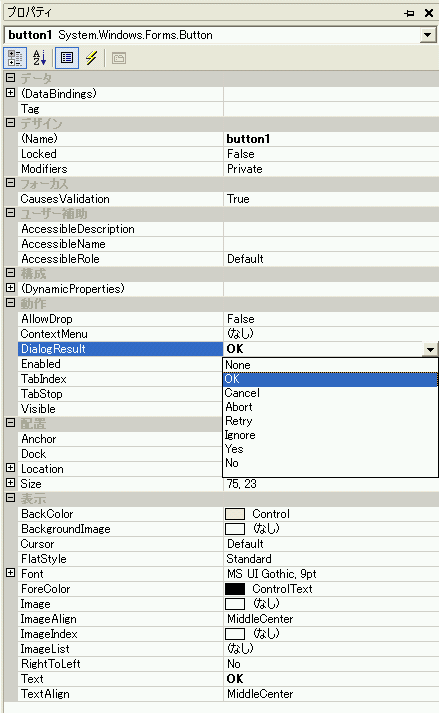 |
5 | ダイアログで リターンキーを押した場合は OK ボタン、エスケープを押した場合は
キャンセルボタンを押したのと同じ効果を得るというWindows のお作法があるようですね。 それを実現するには、FormのAcceptButton と、CancelButton プロパティを使用します。 次のように、Form2 のプロパティを開き、AcceptButton には OKボタン、CancelButton には Cancel
ボタンをそれぞれ割り当てるだけでOKです。
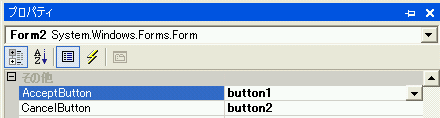
|
6 | 以上で、ほとんど実装することなく、モーダルダイアログができます。 |
|
|
モーダルダイアログでは、ShowDialog() で呼び出しましたが、モードレスダイアログでは Show()
で呼び出します。 Show() の返り値は、void で、先ほどのような DialogResult は使えません。 それでは、先ほどの Form2 のモーダルダイアログをモードレスで呼び出してみましょう。
手順 | 作り方 |
1 | メインウィンドウの Form1.cs にボタンを追加し、モーダルダイアログという名前にします。 |
2 |
1で作ったボタンをダブルクリックし、イベントハンドラを追加します。
private void button2_Click(object sender, System.EventArgs e)
{
Form2 form2 = new Form2();
form2.Show();
}
コンパイルして、モードレスダイアログを1回押してみてください。Form2 が表示されたと思います。 ここで、OK, Cancel などのボタンを押してみてください。今度は、何も起こらないですよね。 Xボタンで、明示的にForm を閉じるまで、モーダルダイアログは存在し続けます。 button2_Click で作った form2 インスタンスは、 どこかメモリ上で宙ぶらりんの状態(ダングリング ポインター)になってしまいます。 ですから、このような実装は NG です。
では、連続してモードレスダイアログのボタンを2回押してみてください。 今度は、モーダルダイアログが2つ表示されたと思います。
このように、何も考えずに form2.Show() をどんどん呼べてしまうので、 モーダルダイアログの場合はインスタンスの管理をしっかりするか、 次のようなシングルトンにしないとバグの原因になります。
|
|
|
たとえば、フォントを設定するようなダイアログは、複数表示したくないですよね。 このような場合には、シングルトン ダイアログにすることにより解決できます。 シングルトンにする方法は、こちらを参照にしてください。
注意: この方法だと、Form を X
ボタンで閉じて、もう一度 Form を起動した場合に、 Form のインスタンスが残っているにもかかわらず Form のコントロールが Dispose されてしまい、 エラーになってしまいます。 このため、次のように Dispose された場合にインスタンスを作り直すようにする必要があります。
手順 | 作り方 |
1 | Form3 をプロジェクトに追加します。
Form3 は、シールドクラスにして次で説明する Instance() をオーバライドされないようにします。
sealed class Form3 : System.Windows.Forms.Form |
2 | Form3 のコンストラクターを private にし、Form
のインスタンスを次のように定義します。
private static Form3 instance = new Form3();
public static Form3 Instance()
{
if (instance.IsDisposed)
instance = new Form3();
return instance;
}
|
3 |
Form1 でボタンが押されたら、次のように Form3 のインスタンスに対し、Show() します。
private void button3_Click(object sender, System.EventArgs e)
{
Form3.Instance().Show();
}
|
|
|
モーダル
フォームの親子関係を持たせることにより、親のフォームを最小化すると、子のフォームを最小化することができます。 また、同様に親フォームを閉じると、子フォームも閉じることができます。
逆に言えば、親子関係がないモーダルフォームは、親を最小化しても、他のモーダルフォームには影響ないということです。 ただし、起動もとのフォームを閉じてしまうと、インスタンスそのものがなくなってしまうので、他のモーダルフォームも存在できなくなり、 すべてのフォームが閉じることになります。
手順 | 作り方 |
自分を子フォームにする | 子になるフォームのプロパティの Owner で親フォームのインスタンスをしてします。 すると、自動的に親フォームの OwnedForms プロパティに子フォームのインスタンスが追加されます。
|
子フォームをやめる。 | フォームのプロパティの Owner を null にする。 |
自分を親フォームにする。 | 親になるフォームの AddOwnedForm(子フォームのインスタンス) メソッドで子フォームを指定します(養子と同じ)。 すると、自動的に子フォームの Owner プロパティに親フォームのインスタンスが追加されます。
|
親フォームをやめる。 | フォームの RemoveOwnedForm(子フォームのインスタンス) で子フォームをはずします(捨て子と同じ)。 すると、自動的に子フォームの Owner プロパティも null になります。 |
|
|
MDI ウィンドウを作るには、まず親ウィンドウの IsMdiContainer プロパティを true にします。 次に、子ウィンドウ側の MdiParent で親ウィンドウを指定します。
手順 | 作り方 |
親ウィンドウから 子ウィンドウをMDI にする。 | private Form form2 = new Form2();
this.IsMdiContainer = true; form2.Show(); form3.Show();
form2.MdiParent = this;
|
子ウィンドウから、 MDI から抜ける。 | this.MdiParent = null; |
|
|
3つのフォーム(Form1.cs, Form2.cs, Form3.cs) から構成されています。
Form1.cs |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Dialogs1
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Button button3;
private System.Windows.Forms.Button button4;
private System.Windows.Forms.Button button5;
private System.Windows.Forms.GroupBox groupBox1;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.label1 = new System.Windows.Forms.Label();
this.button3 = new System.Windows.Forms.Button();
this.button4 = new System.Windows.Forms.Button();
this.button5 = new System.Windows.Forms.Button();
this.groupBox1 = new System.Windows.Forms.GroupBox();
this.groupBox1.SuspendLayout();
this.SuspendLayout();
//
// button1
//
this.button1.Location = new System.Drawing.Point(32, 16);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(176, 40);
this.button1.TabIndex = 3;
this.button1.Text = "モードレス ダイアログ";
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// button2
//
this.button2.Location = new System.Drawing.Point(32, 72);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(176, 40);
this.button2.TabIndex = 4;
this.button2.Text = "モーダル ダイアログ";
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// label1
//
this.label1.Location = new System.Drawing.Point(248, 24);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(176, 23);
this.label1.TabIndex = 5;
this.label1.Text = "ModalDialog の結果";
//
// button3
//
this.button3.Location = new System.Drawing.Point(32, 160);
this.button3.Name = "button3";
this.button3.Size = new System.Drawing.Size(176, 40);
this.button3.TabIndex = 6;
this.button3.Text = "シングルトン モーダル ダイアログ";
this.button3.Click += new System.EventHandler(this.button3_Click);
//
// button4
//
this.button4.Location = new System.Drawing.Point(120, 88);
this.button4.Name = "button4";
this.button4.Size = new System.Drawing.Size(112, 32);
this.button4.TabIndex = 7;
this.button4.Text = "従属にする。";
this.button4.Click += new System.EventHandler(this.button4_Click);
//
// button5
//
this.button5.Location = new System.Drawing.Point(248, 88);
this.button5.Name = "button5";
this.button5.Size = new System.Drawing.Size(112, 32);
this.button5.TabIndex = 8;
this.button5.Text = "従属を解除する。";
this.button5.Click += new System.EventHandler(this.button5_Click);
//
// groupBox1
//
this.groupBox1.Controls.AddRange(new System.Windows.Forms.Control[] {
this.button4,
this.button5});
this.groupBox1.Location = new System.Drawing.Point(16, 128);
this.groupBox1.Name = "groupBox1";
this.groupBox1.Size = new System.Drawing.Size(384, 136);
this.groupBox1.TabIndex = 9;
this.groupBox1.TabStop = false;
this.groupBox1.Text = "groupBox1";
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(432, 278);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.button3,
this.label1,
this.button2,
this.button1,
this.groupBox1});
this.Name = "Form1";
this.Text = "Form1";
this.groupBox1.ResumeLayout(false);
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void button1_Click(object sender, System.EventArgs e)
{
Form modalDialog = new Form2();
DialogResult result = modalDialog.ShowDialog();
switch(result)
{
case DialogResult.OK:
//
break;
case DialogResult.Cancel:
//
break;
case DialogResult.Yes:
//
break;
case DialogResult.No:
//
break;
case DialogResult.Abort:
//
break;
case DialogResult.Ignore:
//
break;
case DialogResult.Retry:
//
break;
case DialogResult.None:
//
break;
}
this.label1.Text = result.ToString() + " が押されました。";
}
private void button2_Click(object sender, System.EventArgs e)
{
Form2 form2 = new Form2();
form2.Show();
}
private void button3_Click(object sender, System.EventArgs e)
{
Form3.Instance().Show();
}
private void button4_Click(object sender, System.EventArgs e)
{
this.AddOwnedForm(Form3.Instance());
}
private void button5_Click(object sender, System.EventArgs e)
{
this.RemoveOwnedForm(Form3.Instance());
}
private void button6_Click(object sender, System.EventArgs e)
{
this.IsMdiContainer = true;
Form3.Instance().MdiParent = this;
}
}
}
|
Form2.cs |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace Dialogs1
{
/// <summary>
/// Form2 の概要の説明です。
/// </summary>
public class Form2 : System.Windows.Forms.Form
{
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.RadioButton radioButton1;
private System.Windows.Forms.RadioButton radioButton2;
private System.Windows.Forms.RadioButton radioButton3;
private System.Windows.Forms.RadioButton radioButton4;
private System.Windows.Forms.RadioButton radioButton5;
private System.Windows.Forms.RadioButton radioButton6;
private System.Windows.Forms.RadioButton radioButton7;
private System.Windows.Forms.Button button3;
private System.Windows.Forms.Button button4;
private System.Windows.Forms.Button button5;
private System.Windows.Forms.Button button6;
private System.Windows.Forms.Button button7;
private System.Windows.Forms.GroupBox groupBox1;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form2()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
// デフォルトで FormBorderStyle は Sizable
this.radioButton5.Checked = true;
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.radioButton1 = new System.Windows.Forms.RadioButton();
this.radioButton2 = new System.Windows.Forms.RadioButton();
this.radioButton3 = new System.Windows.Forms.RadioButton();
this.radioButton4 = new System.Windows.Forms.RadioButton();
this.radioButton5 = new System.Windows.Forms.RadioButton();
this.radioButton6 = new System.Windows.Forms.RadioButton();
this.radioButton7 = new System.Windows.Forms.RadioButton();
this.button3 = new System.Windows.Forms.Button();
this.button4 = new System.Windows.Forms.Button();
this.button5 = new System.Windows.Forms.Button();
this.button6 = new System.Windows.Forms.Button();
this.button7 = new System.Windows.Forms.Button();
this.groupBox1 = new System.Windows.Forms.GroupBox();
this.SuspendLayout();
//
// button1
//
this.button1.DialogResult = System.Windows.Forms.DialogResult.OK;
this.button1.Location = new System.Drawing.Point(200, 8);
this.button1.Name = "button1";
this.button1.TabIndex = 0;
this.button1.Text = "OK";
//
// button2
//
this.button2.DialogResult = System.Windows.Forms.DialogResult.Cancel;
this.button2.Location = new System.Drawing.Point(200, 40);
this.button2.Name = "button2";
this.button2.TabIndex = 1;
this.button2.Text = "Cancel";
//
// radioButton1
//
this.radioButton1.Location = new System.Drawing.Point(24, 32);
this.radioButton1.Name = "radioButton1";
this.radioButton1.TabIndex = 2;
this.radioButton1.Text = "None";
this.radioButton1.CheckedChanged += new System.EventHandler(this.OnCheckedChanged);
//
// radioButton2
//
this.radioButton2.Location = new System.Drawing.Point(24, 64);
this.radioButton2.Name = "radioButton2";
this.radioButton2.TabIndex = 3;
this.radioButton2.Text = "FixedSingle";
this.radioButton2.CheckedChanged += new System.EventHandler(this.OnCheckedChanged);
//
// radioButton3
//
this.radioButton3.Location = new System.Drawing.Point(24, 96);
this.radioButton3.Name = "radioButton3";
this.radioButton3.TabIndex = 4;
this.radioButton3.Text = "Fixed3D";
this.radioButton3.CheckedChanged += new System.EventHandler(this.OnCheckedChanged);
//
// radioButton4
//
this.radioButton4.Location = new System.Drawing.Point(24, 128);
this.radioButton4.Name = "radioButton4";
this.radioButton4.TabIndex = 5;
this.radioButton4.Text = "FixedDialog";
this.radioButton4.CheckedChanged += new System.EventHandler(this.OnCheckedChanged);
//
// radioButton5
//
this.radioButton5.Location = new System.Drawing.Point(24, 160);
this.radioButton5.Name = "radioButton5";
this.radioButton5.TabIndex = 6;
this.radioButton5.Text = "Sizable";
this.radioButton5.CheckedChanged += new System.EventHandler(this.OnCheckedChanged);
//
// radioButton6
//
this.radioButton6.Location = new System.Drawing.Point(24, 192);
this.radioButton6.Name = "radioButton6";
this.radioButton6.Size = new System.Drawing.Size(112, 24);
this.radioButton6.TabIndex = 7;
this.radioButton6.Text = "FixedToolWindow";
this.radioButton6.CheckedChanged += new System.EventHandler(this.OnCheckedChanged);
//
// radioButton7
//
this.radioButton7.Location = new System.Drawing.Point(24, 224);
this.radioButton7.Name = "radioButton7";
this.radioButton7.Size = new System.Drawing.Size(120, 24);
this.radioButton7.TabIndex = 8;
this.radioButton7.Text = "SizableToolWindow";
this.radioButton7.CheckedChanged += new System.EventHandler(this.OnCheckedChanged);
//
// button3
//
this.button3.DialogResult = System.Windows.Forms.DialogResult.Abort;
this.button3.Location = new System.Drawing.Point(200, 80);
this.button3.Name = "button3";
this.button3.TabIndex = 9;
this.button3.Text = "Abort";
//
// button4
//
this.button4.DialogResult = System.Windows.Forms.DialogResult.Yes;
this.button4.Location = new System.Drawing.Point(200, 120);
this.button4.Name = "button4";
this.button4.TabIndex = 10;
this.button4.Text = "Yes";
//
// button5
//
this.button5.DialogResult = System.Windows.Forms.DialogResult.No;
this.button5.Location = new System.Drawing.Point(200, 160);
this.button5.Name = "button5";
this.button5.TabIndex = 11;
this.button5.Text = "No";
//
// button6
//
this.button6.DialogResult = System.Windows.Forms.DialogResult.Retry;
this.button6.Location = new System.Drawing.Point(200, 200);
this.button6.Name = "button6";
this.button6.TabIndex = 12;
this.button6.Text = "Retry";
//
// button7
//
this.button7.DialogResult = System.Windows.Forms.DialogResult.Ignore;
this.button7.Location = new System.Drawing.Point(200, 232);
this.button7.Name = "button7";
this.button7.TabIndex = 13;
this.button7.Text = "Ignore";
//
// groupBox1
//
this.groupBox1.Location = new System.Drawing.Point(8, 8);
this.groupBox1.Name = "groupBox1";
this.groupBox1.Size = new System.Drawing.Size(176, 248);
this.groupBox1.TabIndex = 14;
this.groupBox1.TabStop = false;
this.groupBox1.Text = "FormBorderStyle";
//
// Form2
//
this.AcceptButton = this.button1;
this.AutoScale = false;
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.CancelButton = this.button2;
this.ClientSize = new System.Drawing.Size(292, 266);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.button7,
this.button6,
this.button5,
this.button4,
this.button3,
this.radioButton7,
this.radioButton6,
this.radioButton5,
this.radioButton4,
this.radioButton3,
this.radioButton2,
this.radioButton1,
this.button2,
this.button1,
this.groupBox1});
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog;
this.Name = "Form2";
this.Text = "Form2";
this.ResumeLayout(false);
}
#endregion
private void OnCheckedChanged(object sender, System.EventArgs e)
{
if (this.radioButton1.Checked)
this.FormBorderStyle = FormBorderStyle.None;
else if (this.radioButton2.Checked)
this.FormBorderStyle = FormBorderStyle.FixedSingle;
else if (this.radioButton3.Checked)
this.FormBorderStyle = FormBorderStyle.Fixed3D;
else if (this.radioButton4.Checked)
this.FormBorderStyle = FormBorderStyle.FixedDialog;
else if (this.radioButton5.Checked)
this.FormBorderStyle = FormBorderStyle.Sizable;
else if (this.radioButton6.Checked)
this.FormBorderStyle = FormBorderStyle.FixedToolWindow;
else if (this.radioButton7.Checked)
this.FormBorderStyle = FormBorderStyle.SizableToolWindow;
}
}
}
|
Form3.cs |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace Dialogs1
{
/// <summary>
/// Form3 の概要の説明です。
/// </summary>
sealed class Form3 : System.Windows.Forms.Form
{
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
private Form3()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// button1
//
this.button1.Location = new System.Drawing.Point(40, 64);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(96, 23);
this.button1.TabIndex = 3;
this.button1.Text = "子供をやめる";
this.button1.Click += new System.EventHandler(this.button1_Click_1);
//
// Form3
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(292, 118);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.button1});
this.Name = "Form3";
this.Text = "Form3";
this.ResumeLayout(false);
}
#endregion
private System.Windows.Forms.Button button1;
private static Form3 instance = new Form3();
public static Form3 Instance()
{
if (instance.IsDisposed)
instance = new Form3();
return instance;
}
private void button1_Click_1(object sender, System.EventArgs e)
{
this.Owner = null;
}
}
}
|
|
|
3つのフォーム(Form1.cs, Form2.cs, Form3.cs) から構成されています。
Form1.cs |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Dialog2
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// button1
//
this.button1.Location = new System.Drawing.Point(8, 8);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(152, 23);
this.button1.TabIndex = 0;
this.button1.Text = "Form2 を MDI にする。";
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// button2
//
this.button2.Location = new System.Drawing.Point(8, 40);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(152, 23);
this.button2.TabIndex = 1;
this.button2.Text = "Form3 を MDI にする。";
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(292, 266);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.button2,
this.button1});
this.Name = "Form1";
this.Text = "Form1";
this.Load += new System.EventHandler(this.Form1_Load);
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private Form form2 = new Form2();
private Form form3 = new Form3();
private void Form1_Load(object sender, System.EventArgs e)
{
this.IsMdiContainer = true;
form2.Show();
form3.Show();
}
private void button1_Click(object sender, System.EventArgs e)
{
form2.MdiParent = this;
}
private void button2_Click(object sender, System.EventArgs e)
{
form3.MdiParent = this;
}
}
}
|
Form2.cs |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace Dialog2
{
/// <summary>
/// Form2 の概要の説明です。
/// </summary>
public class Form2 : System.Windows.Forms.Form
{
private System.Windows.Forms.Button button1;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form2()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// button1
//
this.button1.Location = new System.Drawing.Point(40, 32);
this.button1.Name = "button1";
this.button1.TabIndex = 0;
this.button1.Text = "MDI を解除";
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// Form2
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(200, 102);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.button1});
this.Name = "Form2";
this.Text = "Form2";
this.ResumeLayout(false);
}
#endregion
private void button1_Click(object sender, System.EventArgs e)
{
this.MdiParent = null;
}
}
}
|
Form3.cs |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace Dialog2
{
/// <summary>
/// Form3 の概要の説明です。
/// </summary>
public class Form3 : System.Windows.Forms.Form
{
private System.Windows.Forms.Button button1;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form3()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// button1
//
this.button1.Location = new System.Drawing.Point(8, 8);
this.button1.Name = "button1";
this.button1.TabIndex = 0;
this.button1.Text = "MDI を解除";
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// Form3
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(192, 78);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.button1});
this.Name = "Form3";
this.Text = "Form3";
this.ResumeLayout(false);
}
#endregion
private void button1_Click(object sender, System.EventArgs e)
{
this.MdiParent = null;
}
}
}
|
|