デジタルクロックを作る (その2)
開発環境: Visual Studio 2003
次のような機能のForm アプリケーションを作る。
作り方
注意 デジタルクロックを作る(その1)と違う部分を 赤で 記述
1. まず フォームの BackgroundImage プロパティに、適当な画像を貼り付ける。
2. フォームにツールボックスより、タイマーを貼り付ける。
3. TextBox のプロパティ、Form のプロパティを次のようにセットする。
デジタルクロックを作る(その1)と違う部分は、赤の部分。
Formのプロパティ
プロパティ |
値 |
Text |
C# Digital Clock |
TextBoxのプロパティ
プロパティ |
値 |
font |
36ポイント(任意) |
ForeColor |
Lime(任意) |
BackColor |
ブラック(任意) |
TextAlign |
Center |
Dock |
None |
Cursor |
No |
Anchor |
Top, Left |
ReadOnly |
True |
BackgoundImage |
xxxx.gif(任意) |
Timerのプロパティ
プロパティ |
値 |
Enabled |
True |
Interval |
1000 |
ここまでくれば、次のようなフォームデザインになるはず。
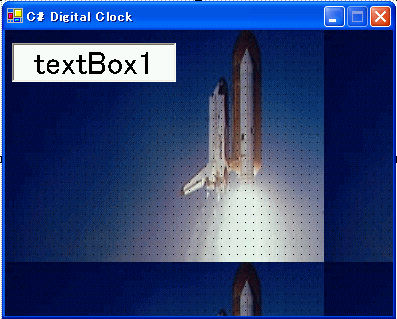
5. これを見ればわかるように、バックグランド画像はタイル状に表示されてしまう。
そこで、Form のバックグラウンドにあわせて Form をリサイズするメソッドを手で追加します。
private void FitToBackgroundImage()
{
this.SetClientSizeCore(this.BackgroundImage.Width,
this.BackgroundImage.Height);
}
次に、フォームをダブルクリックしてください。
すると、自動的に MyClock_Loadイベントハンドラが追加されて、コードが表示されます。
そこで、FitToBackgroundImage()を呼び出すようにコードを追加します。
private void MyClock_Load(object sender, System.EventArgs e)
{
this.FitToBackgroundImage();
}
6. あとは、Timer のプロパティより、Tick イベントに OnTick をタイプすると、自動的にイベントが追加され、コードが表示される。
あとは、イベント発生時に、テキストボックスのTextプロパティに時間をセットすればよい。
コードを追加する必要があるのは、次の1行だけ。
private void OnTick(object sender, System.EventArgs e)
{
timeTextBox.Text = DateTime.Now.ToLongTimeString();
}
日付 |
修正履歴 |
2002/4/28 | バックグラウンドイメージの大きさに合わせて、自動的にウィンドウをリサイズするように修正。 |
Timer コンポーネントではなく、Timer コントロールを使用するように修正。 |
2003/12/7 | VS.2003、.NetFramework V1.1 に対応。 |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace MyClock
{
/// <summary>
/// MyClockです。
/// </summary>
public class MyClock : System.Windows.Forms.Form
{
private System.Windows.Forms.Timer timer1;
private System.Windows.Forms.TextBox textBox1;
private System.ComponentModel.IContainer components;
public MyClock()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
this.FitToBackgroundImage();
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要です。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
System.Resources.ResourceManager resources = new System.Resources.ResourceManager(typeof(MyClock));
this.timer1 = new System.Windows.Forms.Timer(this.components);
this.textBox1 = new System.Windows.Forms.TextBox();
this.SuspendLayout();
//
// timer1
//
this.timer1.Enabled = true;
this.timer1.Interval = 1000;
this.timer1.Tick += new System.EventHandler(this.OnTick);
//
// textBox1
//
this.textBox1.BackColor = System.Drawing.Color.Black;
this.textBox1.font = new System.Drawing.font("MS UI Gothic", 24F, System.Drawing.fontStyle.Regular,
System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.textBox1.ForeColor = System.Drawing.Color.Lime;
this.textBox1.Location = new System.Drawing.Point(7, 13);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(164, 39);
this.textBox1.TabIndex = 1;
this.textBox1.Text = "";
this.textBox1.TextAlign = System.Windows.Forms.HorizontalAlignment.Center;
//
// MyClock
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.BackgroundImage = ((System.Drawing.Bitmap)(resources.GetObject("$this.BackgroundImage")));
this.ClientSize = new System.Drawing.Size(388, 286);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.textBox1});
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedSingle;
this.MaximizeBox = false;
this.Name = "MyClock";
this.Text = "C# Digital Clock";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new MyClock());
}
private void OnTick(object sender, System.EventArgs e)
{
this.textBox1.Text = DateTime.Now.ToLongTimeString();
}
private void FitToBackgroundImage()
{
this.SetClientSizeCore(this.BackgroundImage.Width, this.BackgroundImage.Height);
}
}
}