|

フォルダーを選択するダイアログ(VisualBasicコンパチ)
|
開発環境: Visual Studio 2002
.NET Framework V1.0
では、フォルダを選択するダイアログがサポートされておらず、 自分で作るとか、未サポートのメソッドを使うとか、VBコンパチライブラリを使う必要がありました。
.Net Framework V1.1 以降では、FolderBrowserDialog が標準でサポートされるようになり、楽になっています。
以下の記事は、V1.0の名残なので、参考程度にしてください。 |
Microsoft.VisualBasic.Compatibility.VB6.DirListBox
Microsoft.VisualBasic.Compatibility.VB6.DriveListBox
注意: C#.Std では、DirListBox, DriveListBox がバンドルされていないので、使えないようです。 |
次のような機能のForm アプリケーションを作る。
- ディレクトリを選択し、TextBox に表示する。
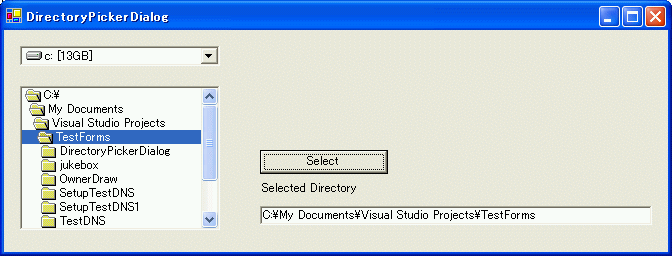
Microsoft.VisualBasic.Compatibility.VB6.DirListBox
Microsoft.VisualBasic.Compatibility.VB6.DriveListBox
が用意されており、一応 VB6
用のコンパチビリティのために用意されているコントロールを使うことができる。
しかし、標準ではツールボックスには表示されない。
そこで、次の図のようにツールボックス上で右ボタンを押すと、ツールボックスのカスタマイズというメニューがある。
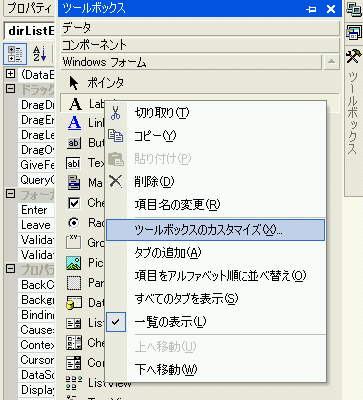
これを選択すると、次のようなツールボックスのカスタマイズダイアログが表示されるので、
.NET Framework コンポーネントから、
Microsoft.VisualBasic.Compatibility.VB6.DirListBox
Microsoft.VisualBasic.Compatibility.VB6.DriveListBox
のチェックボックスを ON にする。
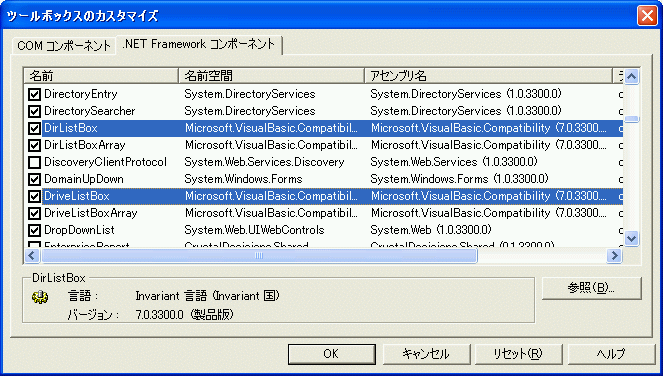
これにより、次のように DirListBox, DriveListBox がツールボックス上に表示されるようになる。
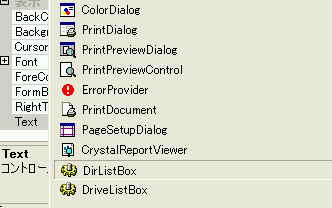
あとは、普通に書けばなんとかなるでしょう。
コードを書く必要があるのは、これだけ。
private void OnMouseDown(object sender, System.Windows.Forms.MouseEventArgs e)
{
this.textBox1.Text = this.dirListBox1.Path;
}
private void OnDirSelectChanged(object sender, System.EventArgs e)
{
try
{
this.dirListBox1.Path = this.driveListBox1.Drive;
}
catch(System.Exception exc)
{
// ドライブが準備できていないなどのエラーの例外処理
MessageBox.Show(exc.Message, this.Text, MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
}
}
VB6 用のコンパチビリティのためだし、またメニューからも隠してあるので、MSの保証の限りではないでしょう。
以下ソースコード。
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace DirectoryPickerDialog
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class DirectoryPickerDialog : System.Windows.Forms.Form
{
private System.Windows.Forms.OpenFileDialog openFileDialog1;
private System.Windows.Forms.Button button1;
private Microsoft.VisualBasic.Compatibility.VB6.DirListBox dirListBox1;
private Microsoft.VisualBasic.Compatibility.VB6.DriveListBox driveListBox1;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.Label label1;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public DirectoryPickerDialog()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
System.Configuration.AppSettingsReader configurationAppSettings = new System.Configuration.AppSettingsReader();
this.openFileDialog1 = new System.Windows.Forms.OpenFileDialog();
this.button1 = new System.Windows.Forms.Button();
this.dirListBox1 = new Microsoft.VisualBasic.Compatibility.VB6.DirListBox();
this.driveListBox1 = new Microsoft.VisualBasic.Compatibility.VB6.DriveListBox();
this.textBox1 = new System.Windows.Forms.TextBox();
this.label1 = new System.Windows.Forms.Label();
this.SuspendLayout();
//
// openFileDialog1
//
this.openFileDialog1.Filter = "aaa|*|aaaa|*.*";
//
// button1
//
this.button1.Location = new System.Drawing.Point(256, 120);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(128, 24);
this.button1.TabIndex = 0;
this.button1.Text = "Select";
this.button1.MouseDown += new System.Windows.Forms.MouseEventHandler(this.OnMouseDown);
//
// dirListBox1
//
this.dirListBox1.IntegralHeight = false;
this.dirListBox1.Location = new System.Drawing.Point(16, 56);
this.dirListBox1.Name = "dirListBox1";
this.dirListBox1.Size = new System.Drawing.Size(200, 144);
this.dirListBox1.TabIndex = 1;
//
// driveListBox1
//
this.driveListBox1.Location = new System.Drawing.Point(16, 16);
this.driveListBox1.Name = "driveListBox1";
this.driveListBox1.Size = new System.Drawing.Size(200, 20);
this.driveListBox1.TabIndex = 2;
this.driveListBox1.SelectedIndexChanged += new System.EventHandler(this.OnDirSelectChanged);
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(256, 176);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(392, 19);
this.textBox1.TabIndex = 3;
this.textBox1.Text = ((string)(configurationAppSettings.GetValue("textBox1.Text", typeof(string))));
//
// label1
//
this.label1.Location = new System.Drawing.Point(256, 152);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(112, 16);
this.label1.TabIndex = 4;
this.label1.Text = "Selected Directory";
//
// DirectoryPickerDialog
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(664, 222);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.label1,
this.textBox1,
this.driveListBox1,
this.dirListBox1,
this.button1});
this.Name = "DirectoryPickerDialog";
this.Text = "DirectoryPickerDialog";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new DirectoryPickerDialog());
}
private void OnMouseDown(object sender, System.Windows.Forms.MouseEventArgs e)
{
this.textBox1.Text = this.dirListBox1.Path;
}
private void OnDirSelectChanged(object sender, System.EventArgs e)
{
try
{
this.dirListBox1.Path = this.driveListBox1.Drive;
}
catch(System.Exception exc)
{
// ドライブが準備できていないなどのエラーの例外処理
MessageBox.Show(exc.Message, this.Text, MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
}
}
}
}