|

ErrorProvider
|
開発環境: Visual Studio 2003
次のような機能のアプリケーションを作る。
・数字を入力するフィールド(NumericUpDown。
・ただし、偶数でない場合は ErrorProvider を表示する。
・TextBox。先頭がAでない場合は Error Provider を表示する。
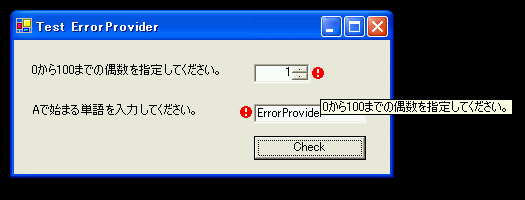
作り方
1. ツールボックスより、NumericUpDown コントロールを貼り付ける。
2. ツールボックスより、TextBox コントロールを貼り付ける。
3. ツールボックスより、Button コントロールを貼り付け、Textプロパティを "Check"に変更する。
4. ツールボックスより、ErrorProvider コントロールを貼り付ける。
5. Checkボタンを押したときのイベントハンドラを追加する。
private void OnCheck(object sender, System.EventArgs e)
{
if (this.numericUpDown1.Value % 2 != 0)
{
this.errorProvider1.SetError(this.numericUpDown1, this.label1.Text);
}
else
{
this.errorProvider1.SetError(this.numericUpDown1, null);
}
if (this.textBox1.Text.Length == 0 || this.textBox1.Text[0] != 'A')
{
this.errorProvider1.SetIconAlignment(this.textBox1, System.Windows.Forms.ErrorIconAlignment.TopLeft);
this.errorProvider1.SetError((this.textBox1, this.label2.Text);
}
else
{
this.errorProvider1.SetError(this.textBox1, null);
}
}
6. この中で条件をチェックし、エラーの場合には ErrorProvider.SetError(...) を呼び出す。
ここで、ErrorProvider.SetError( エラープロバイダを貼り付けるコントロール, エラーを説明する文字列);
BlinkRate、BlinkStyle, Icon プロパティにより、アイコンの点滅間隔、アイコンの表示位置、アイコン図形を変更することができる。
これ以外に、ADO.Net データベースを処理する場合に、DataSource と DataMember を設定する方法もある。
ソースコードは次のとおり。
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace ErrorProvider
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.ErrorProvider errorProvider1;
private System.Windows.Forms.NumericUpDown numericUpDown1;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label2;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.errorProvider1 = new System.Windows.Forms.ErrorProvider();
this.numericUpDown1 = new System.Windows.Forms.NumericUpDown();
this.textBox1 = new System.Windows.Forms.TextBox();
this.button1 = new System.Windows.Forms.Button();
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
((System.ComponentModel.ISupportInitialize)(this.numericUpDown1)).BeginInit();
this.SuspendLayout();
//
// errorProvider1
//
this.errorProvider1.DataMember = null;
//
// numericUpDown1
//
this.numericUpDown1.Location = new System.Drawing.Point(240, 24);
this.numericUpDown1.Name = "numericUpDown1";
this.numericUpDown1.Size = new System.Drawing.Size(56, 19);
this.numericUpDown1.TabIndex = 0;
this.numericUpDown1.TextAlign = System.Windows.Forms.HorizontalAlignment.Right;
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(240, 64);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(112, 19);
this.textBox1.TabIndex = 1;
this.textBox1.Text = "";
//
// button1
//
this.button1.Location = new System.Drawing.Point(240, 96);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(112, 24);
this.button1.TabIndex = 2;
this.button1.Text = "Check";
this.button1.Click += new System.EventHandler(this.OnCheck);
//
// label1
//
this.label1.Location = new System.Drawing.Point(16, 24);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(200, 24);
this.label1.TabIndex = 3;
this.label1.Text = "0から100までの偶数を指定してください。";
//
// label2
//
this.label2.Location = new System.Drawing.Point(16, 64);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(200, 24);
this.label2.TabIndex = 4;
this.label2.Text = "Aで始まる単語を入力してください。";
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(376, 134);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.label2,
this.label1,
this.button1,
this.textBox1,
this.numericUpDown1});
this.Name = "Form1";
this.Text = "Test ErrorProvider";
((System.ComponentModel.ISupportInitialize)(this.numericUpDown1)).EndInit();
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void OnCheck(object sender, System.EventArgs e)
{
if (this.numericUpDown1.Value % 2 != 0)
{
this.errorProvider1.SetError(this.numericUpDown1, this.label1.Text);
}
else
{
this.errorProvider1.SetError(this.numericUpDown1, null);
}
if (this.textBox1.Text.Length == 0 || this.textBox1.Text[0] != 'A')
{
this.errorProvider1.SetIconAlignment(this.textBox1, System.Windows.Forms.ErrorIconAlignment.TopLeft);
this.errorProvider1.SetError((this.textBox1, this.label2.Text);
}
else
{
this.errorProvider1.SetError(this.textBox1, null);
}
}
}
}