C# RegEx 正規表現
開発環境: Visual Studio 2003-2010
1.目次
1.目次
2.目的
3.参考書
4.超簡易正規表現作成支援ツール
5.よく使う正規表現の例
6.よく使う正規表現
5.RegEx デザインパターン
7.ダウンロード
8.ソースコード
2.目的
たま〜〜に、正規表現が必要になることがあるんですけど、たまにしか使わないからすぐ忘れちゃうんですよね。
今回もたまたま正規表現が必要になる場面があったんですが、使おうと思ってもヘルプの場所を探すのでひと苦労。
それに正規表現が正しいかどうかプログラム内で確認しようとすると、これがまたやっかい!
いいかげんいやになって、超簡易正規表現作成支援ツールを作りました。
Web からの正規表現テストツール
3.参考書
(1) MSDN
正規表現言語要素
(2) MSDN RegEx
(3) C#プログラミング バリデーションへの応用例
4.超簡易正規表現作成支援ツール
使い方は説明要らないと思います。^^;
Test ボタンで IsMatch(), Matches() をテストします。
Replace ボタンで Replace 文字列でマッチするパターンを置換します。
一番下のListView には、Matches() の結果をリストで表示します。
ヘルプからは、リファレンスへ飛べるようにしておきました。
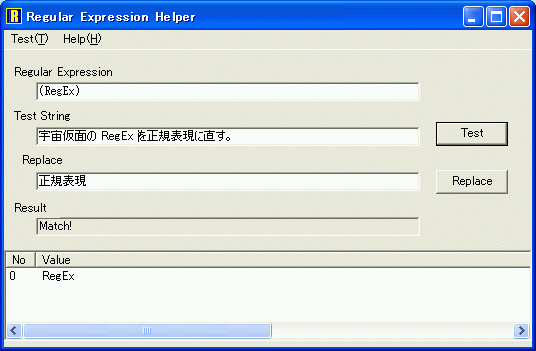
5.よく使う正規表現の例
よく使う正規表現 |
a-z のみの文字列 | @"^[a-z]+$" |
0-9 のみの文字列 | @"^[0-9]+$" |
郵便番号 | @"[0-9]{3}-[0-9]{4}" |
全角ひらがなのみの文字列 | @"^[あ-を]+$" |
募集中^^/ | 募集中^^/ |
6.よく使う正規表現
自分が使うものしか書いてません。
MSDN
正規表現言語要素を参照してね。
アトミック ゼロ幅アサーション |
^ | 文字列の先頭で一致 | ^abc="abc....." |
\A | 文字列の先頭で一致
Multiline オプションを無視 | ^abc="abc....." |
$ | 文字列の末尾で一致 | $abc=".....abc" |
\Z | 文字列の末尾で一致
Multiline オプションを無視 | $abc=".....abc" |
量指定子 |
* | ゼロ回以上の一致
{0,} と同じ | (abc)* = "", abc, abcabc
a* = "", a, aa, aaa |
+ | 1回以上の一致
{1,} と同じ | (abc)+ = abc, abcabc
a+ = a, aa, aaa |
? | ゼロ回または 1 回の一致
{0,1} と同じ | (abc)?="", abc
a?= "" | a |
{n} | 正確に n 回一致 | a{3}= aaa
(pizza){2}=pizzapizza, 123pizzapizzaabc...マッチする |
{n,} | 少なくとも n 回の一致 | (abc){2,} = abcabc, abcabcabc, .... |
{n,m} | n 回以上 m 回以下の一致 | (abc){2,3} = abcabc, abcabcabc |
*? | できるだけ少ない繰り返しを処理する最初の一致 | |
+? | 1 回以上でできるだけ少ない繰り返し | |
?? | できればゼロ回、できない場合は 1 回の繰り返し | |
{n}? | {n} と同じ | |
{n,}? | n 回以上でできるだけ少ない繰り返し | |
{n,m}? | n 回以上 m 回以下でできるだけ少ない繰り返し | |
グループ化構成体 |
( ) | 一致した部分文字列。1 から始まる番号が付けられる。
0番目は、正規表現パターン全体と一致。 | |
(?<name> ) | 一致した部分文字列をグループ名または番号名にキャプチャ
name に使用する文字列は、区切り記号を含まず、先頭が数字以外。 | |
(?'name' ) | 同上。 | |
(?<name1-name2> ) | よくわからん | |
(?: ) | キャプチャしないグループ | |
(?imnsx-imnsx: ) | よくわからん | |
(?= ) | 部分式がこの位置の右で一致した場合にだけ照合を継続 | \w+(?=\d) = abc123 |
(?! ) | 部分式がこの位置の右で一致しない場合にだけ照合を継続 | \b(?!un)\w+\b = subscribe...マッチする
unsubscribe...マッチしない。 |
(?<= ) | 部分式がこの位置の左で一致した場合にだけ照合を継続 | (?<=19)99 = 1999 |
(?<! ) | 部分式がこの位置の左で一致しない場合にだけ照合を継続 | (?<!=19)99 = 1899...マッチする。(?<!=19)99 = 1899...マッチする。
1999...マッチしない。 |
(?> ) | その部分式単独で一致する文字列にだけ一致 | |
前方参照構成体 |
\number | 前方参照 | (\w)\1 重複した単語の文字を検索 |
\k<name> | 名前付き前方参照 | ?<char>\w)\k<char> は、重複した単語の文字を検索 |
代替構成体 |
| | 縦棒で区切られた単語のいずれかと一致
最も左で一致したものが優先 | cat|dog|tiger ...cat, dog, tiger |
(?(expression)yes|no) | この位置で式が一致した場合は、"yes" が一致。
それ以外の場合は "no" 部分が一致。
"no" 部分は省略可。 "no" 部分は省略可。 | |
(?(name)yes|no) | 名前付きキャプチャ文字列が一致した場合は、"yes"が一致。
それ以外は "no"が一致。"no" 部分は省略可。 | |
その他の構成体 |
(?imnsx-imnsx) | よくわからん | |
(?# ) | インライン コメント | |
# | 行末までコメントにする
x オプションまたは RegexOptions.IgnorePatternWhitespace オプションを有効にする必要がある。 | |
5.RegEx デザインパターン
ここで、テストした正規表現は、次のようなパターンで実行できます。
マッチするかどうか確認する。 |
using System.Text.RegularExpressions;
Regex regex = new System.Text.RegularExpressions.Regex("ここに正規表現");
if ( regex.IsMatch("テストする文字列"))
マッチ!
MatchCollection matchCol = regex.Matches(this.tbInput.Text);
for(int i = 0; i < matchCol.Count; i++)
{
matchCol[i].Value);
} |
マッチする文字列を切り出す。 |
using System.Text.RegularExpressions;
Regex regex = new System.Text.RegularExpressions.Regex("ここに正規表現");
MatchCollection matchCol = regex.Matches("テストする文字列");
for(int i = 0; i < matchCol.Count; i++)
{
xxx = matchCol[i].Value);
}
// あるいは
foreach (Match match in matchCol)
{
xxx = match
} |
マッチする文字列を置換する。 |
using System.Text.RegularExpressions;
Regex regex = new System.Text.RegularExpressions.Regex("ここに正規表現");
string replacedString = regex.Replace("置換される文字列",
"置換する文字列"); |
7.ダウンロード
実行ファイル |
.NET Framework 1.0 SP2対応 |
2003/1/23 公開。 |
.NET Framework 3.5対応 |
2009/3/15 公開 |
.NET Framework 4.0対応 |
2010/4/1 |
マルチライン対応 |
2011/1/26
RegExHelper.exe |
RegExHelper V3.5
インストールイメージ |
.NET Framework 1.0 SP2対応 | 2003/1/23
公開。 |
.NET Framework 3.5対応 | 2009/3/15 |
.NET Framework 4.0対応 | 2010/4/1 |
マルチライン対応 | 2011/1/26
RegExHelperSetup.msi |
8.ソースコード
2003/1/23 初版作成。
RegExHelper.cs |
このファイル以外にもインストーラ部分、構成ファイル、About.cs などがありますが、
たいした内容ではないので、このファイルだけです。
このファイルもたいした内容ではありませんが^^; |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Text.RegularExpressions;
namespace RegExHelper
{
/// <summary>
/// 正規表現ヘルパー
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.TextBox tbInput;
private System.Windows.Forms.TextBox tbResult;
private System.Windows.Forms.TextBox tbRegEx;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.ListView listView1;
private System.Windows.Forms.ColumnHeader columnHeader1;
private System.Windows.Forms.ColumnHeader columnHeader2;
private System.Windows.Forms.Button btnTest;
private System.Windows.Forms.TextBox tbReplace;
private System.Windows.Forms.Label label4;
private System.Windows.Forms.Button btnReplace;
private System.Windows.Forms.MainMenu mainMenu1;
private System.Windows.Forms.MenuItem menuItemTest;
private System.Windows.Forms.MenuItem menuItemDoTest;
private System.Windows.Forms.MenuItem menuItemDoReplace;
private System.Windows.Forms.MenuItem menuItemHelp;
private System.Windows.Forms.MenuItem menuItemHelpMSDN;
private System.Windows.Forms.MenuItem menuItemHelpUchukamen;
private System.Windows.Forms.MenuItem menuItemAbout;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.tbInput = new System.Windows.Forms.TextBox();
this.btnTest = new System.Windows.Forms.Button();
this.tbResult = new System.Windows.Forms.TextBox();
this.tbRegEx = new System.Windows.Forms.TextBox();
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.listView1 = new System.Windows.Forms.ListView();
this.columnHeader1 = new System.Windows.Forms.ColumnHeader();
this.columnHeader2 = new System.Windows.Forms.ColumnHeader();
this.tbReplace = new System.Windows.Forms.TextBox();
this.label4 = new System.Windows.Forms.Label();
this.btnReplace = new System.Windows.Forms.Button();
this.mainMenu1 = new System.Windows.Forms.MainMenu();
this.menuItemTest = new System.Windows.Forms.MenuItem();
this.menuItemDoTest = new System.Windows.Forms.MenuItem();
this.menuItemDoReplace = new System.Windows.Forms.MenuItem();
this.menuItemHelp = new System.Windows.Forms.MenuItem();
this.menuItemHelpMSDN = new System.Windows.Forms.MenuItem();
this.menuItemHelpUchukamen = new System.Windows.Forms.MenuItem();
this.menuItemAbout = new System.Windows.Forms.MenuItem();
this.SuspendLayout();
//
// tbInput
//
this.tbInput.Anchor = ((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right);
this.tbInput.Location = new System.Drawing.Point(32, 77);
this.tbInput.Name = "tbInput";
this.tbInput.Size = new System.Drawing.Size(384, 19);
this.tbInput.TabIndex = 3;
this.tbInput.Text = "";
//
// btnTest
//
this.btnTest.Anchor = (System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right);
this.btnTest.Location = new System.Drawing.Point(432, 72);
this.btnTest.Name = "btnTest";
this.btnTest.Size = new System.Drawing.Size(72, 24);
this.btnTest.TabIndex = 6;
this.btnTest.Text = "Test";
this.btnTest.Click += new System.EventHandler(this.OnTest);
//
// tbResult
//
this.tbResult.Anchor = ((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right);
this.tbResult.Location = new System.Drawing.Point(32, 167);
this.tbResult.Name = "tbResult";
this.tbResult.ReadOnly = true;
this.tbResult.Size = new System.Drawing.Size(384, 19);
this.tbResult.TabIndex = 5;
this.tbResult.Text = "";
//
// tbRegEx
//
this.tbRegEx.Anchor = ((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right);
this.tbRegEx.Font = new System.Drawing.Font("MS 明朝", 9F, System.Drawing.FontStyle.Regular,
System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.tbRegEx.Location = new System.Drawing.Point(32, 32);
this.tbRegEx.Name = "tbRegEx";
this.tbRegEx.Size = new System.Drawing.Size(384, 19);
this.tbRegEx.TabIndex = 1;
this.tbRegEx.Text = "";
//
// label1
//
this.label1.Location = new System.Drawing.Point(8, 60);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(72, 16);
this.label1.TabIndex = 2;
this.label1.Text = "Test String";
//
// label2
//
this.label2.Location = new System.Drawing.Point(8, 16);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(124, 16);
this.label2.TabIndex = 0;
this.label2.Text = "Regular Expression";
//
// label3
//
this.label3.Location = new System.Drawing.Point(8, 152);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(48, 16);
this.label3.TabIndex = 4;
this.label3.Text = "Result";
//
// listView1
//
this.listView1.Anchor = (((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right);
this.listView1.Columns.AddRange(new System.Windows.Forms.ColumnHeader[] {
this.columnHeader1,
this.columnHeader2});
this.listView1.Location = new System.Drawing.Point(0, 200);
this.listView1.Name = "listView1";
this.listView1.Size = new System.Drawing.Size(528, 232);
this.listView1.TabIndex = 7;
this.listView1.View = System.Windows.Forms.View.Details;
//
// columnHeader1
//
this.columnHeader1.Text = "No";
this.columnHeader1.Width = 30;
//
// columnHeader2
//
this.columnHeader2.Text = "Value";
this.columnHeader2.Width = 1000;
//
// tbReplace
//
this.tbReplace.Anchor = ((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right);
this.tbReplace.Location = new System.Drawing.Point(32, 122);
this.tbReplace.Name = "tbReplace";
this.tbReplace.Size = new System.Drawing.Size(384, 19);
this.tbReplace.TabIndex = 10;
this.tbReplace.Text = "";
//
// label4
//
this.label4.Location = new System.Drawing.Point(16, 104);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(48, 16);
this.label4.TabIndex = 11;
this.label4.Text = "Replace";
//
// btnReplace
//
this.btnReplace.Anchor = (System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right);
this.btnReplace.Location = new System.Drawing.Point(432, 120);
this.btnReplace.Name = "btnReplace";
this.btnReplace.Size = new System.Drawing.Size(72, 24);
this.btnReplace.TabIndex = 12;
this.btnReplace.Text = "Replace";
this.btnReplace.Click += new System.EventHandler(this.OnReplace);
//
// mainMenu1
//
this.mainMenu1.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.menuItemTest,
this.menuItemHelp});
//
// menuItemTest
//
this.menuItemTest.Index = 0;
this.menuItemTest.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.menuItemDoTest,
this.menuItemDoReplace});
this.menuItemTest.Text = "Test(&T)";
//
// menuItemDoTest
//
this.menuItemDoTest.Index = 0;
this.menuItemDoTest.Text = "Test(&T)";
this.menuItemDoTest.Click += new System.EventHandler(this.OnTest);
//
// menuItemDoReplace
//
this.menuItemDoReplace.Index = 1;
this.menuItemDoReplace.Text = "Replace(&R)";
this.menuItemDoReplace.Click += new System.EventHandler(this.OnReplace);
//
// menuItemHelp
//
this.menuItemHelp.Index = 1;
this.menuItemHelp.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.menuItemHelpMSDN,
this.menuItemHelpUchukamen,
this.menuItemAbout});
this.menuItemHelp.Text = "Help(&H)";
//
// menuItemHelpMSDN
//
this.menuItemHelpMSDN.Index = 0;
this.menuItemHelpMSDN.Text = "MSDN Help(&M)";
this.menuItemHelpMSDN.Click += new System.EventHandler(this.OnHelpMSDN);
//
// menuItemHelpUchukamen
//
this.menuItemHelpUchukamen.Index = 1;
this.menuItemHelpUchukamen.Text = "Uchukamen(&U)";
this.menuItemHelpUchukamen.Click += new System.EventHandler(this.OnHelpUchukamen);
//
// menuItemAbout
//
this.menuItemAbout.Index = 2;
this.menuItemAbout.Text = "Version(&A)";
this.menuItemAbout.Click += new System.EventHandler(this.OnAbout);
//
// Form1
//
this.AcceptButton = this.btnTest;
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(528, 438);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.btnReplace,
this.label4,
this.tbReplace,
this.listView1,
this.label3,
this.label2,
this.label1,
this.tbRegEx,
this.tbResult,
this.btnTest,
this.tbInput});
this.Menu = this.mainMenu1;
this.Name = "Form1";
this.Text = "Regular Expression Helper";
this.Load += new System.EventHandler(this.Form1_Load);
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void OnTest(object sender, System.EventArgs e)
{
try
{
Regex regex = new System.Text.RegularExpressions.Regex(this.tbRegEx.Text);
if ( regex.IsMatch(this.tbInput.Text))
this.tbResult.Text = "Match!";
else
this.tbResult.Text = "Not Match!";
this.listView1.Items.Clear();
MatchCollection matchCol = regex.Matches(this.tbInput.Text);
for(int i = 0; i < matchCol.Count; i++)
{
ListViewItem item = this.listView1.Items.Add(i.ToString());
item.SubItems.Add(matchCol[i].Value);
}
}
catch (Exception exc)
{
this.tbResult.Text = "Error";
MessageBox.Show(exc.Message,
"Regular Expression Helper",
MessageBoxButtons.OK,
MessageBoxIcon.Error);
}
}
private void Form1_Load(object sender, System.EventArgs e)
{
this.Icon = new Icon(GetType(), "App.ico");
}
private void OnReplace(object sender, System.EventArgs e)
{
try
{
this.listView1.Items.Clear();
Regex regex = new System.Text.RegularExpressions.Regex(this.tbRegEx.Text);
this.tbResult.Text = regex.Replace(this.tbInput.Text, this.tbReplace.Text);
}
catch (Exception exc)
{
this.tbResult.Text = "Error";
MessageBox.Show(exc.Message,
"Regular Expression Helper",
MessageBoxButtons.OK,
MessageBoxIcon.Error);
}
}
/// <summary>
/// MSDN Help
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void OnHelpMSDN(object sender, System.EventArgs e)
{
System.Diagnostics.Process.Start(@"http://www.microsoft.com/japan/msdn/library/default.asp?
url=/japan/msdn/library/ja/cpgenref/html/cpconatomiczero-widthassertions.asp");
}
/// <summary>
/// Uchukamen RegEx Support Page
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void OnHelpUchukamen(object sender, System.EventArgs e)
{
System.Diagnostics.Process.Start(@"http://ukamen.hp.infoseek.co.jp/Programming1/RegEx/index.htm");
}
/// <summary>
/// About Dialog
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void OnAbout(object sender, System.EventArgs e)
{
About about = new About();
about.Show();
}
}
}