TabControl, TabPage
開発環境: Visual Studio 2003
TabControl のAlignment
Top
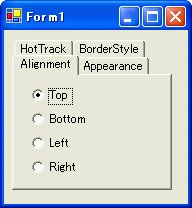
Bottom
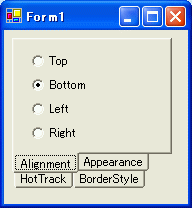
Left
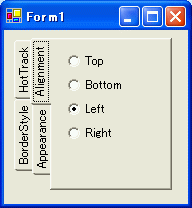
Right
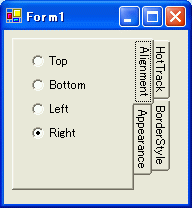
TabControl のAppearance
Normal
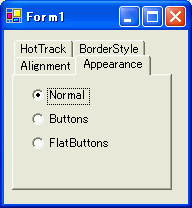
Buttonss
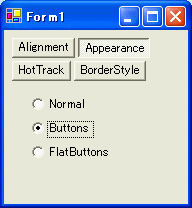
FlatButtons
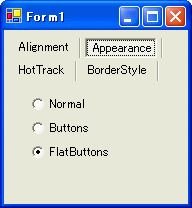
TabControl のHotTrack
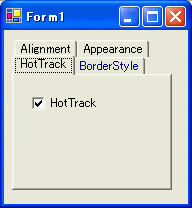
TabPanel のBorderStyle
None
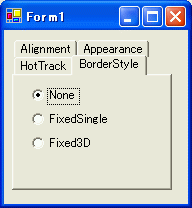
FixedSinglee
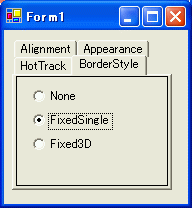
Fixed3D
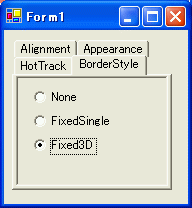
作り方
- 新しいプロジェクト→ Windows アプリケーションを新規作成。
- ツールバーより TabControl を貼り付ける。
- TabControl のプロパティを開き、TabPages コレクションの ... ボタンを押し、TabPage コレクションエディタを開く
。
ここで下図のように追加ボタンを押し、TabPage インスタンスを追加する。
- あとは、TabControl, TabPage のプロパティを適当にセットします。
以下、動作確認用テストツール
日付 | 修正履歴 |
2002/6/29 | 初期バージョン作成 |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace TabControl
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.TabControl tabControl1;
private System.Windows.Forms.TabPage tabPage1;
private System.Windows.Forms.TabPage tabPage2;
private System.Windows.Forms.RadioButton rbTop;
private System.Windows.Forms.RadioButton rbBottom;
private System.Windows.Forms.RadioButton rbLeft;
private System.Windows.Forms.RadioButton rbRight;
private System.Windows.Forms.RadioButton rbNormal;
private System.Windows.Forms.RadioButton rbButtons;
private System.Windows.Forms.RadioButton rbFlatButtons;
private System.Windows.Forms.TabPage tabPage3;
private System.Windows.Forms.CheckBox cbHotTrack;
private System.Windows.Forms.RadioButton rbNone;
private System.Windows.Forms.RadioButton rbFixedSingle;
private System.Windows.Forms.RadioButton rbFixed3D;
private System.Windows.Forms.TabPage tabPageBorderStyle;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.tabControl1 = new System.Windows.Forms.TabControl();
this.tabPage1 = new System.Windows.Forms.TabPage();
this.tabPage2 = new System.Windows.Forms.TabPage();
this.rbTop = new System.Windows.Forms.RadioButton();
this.rbBottom = new System.Windows.Forms.RadioButton();
this.rbLeft = new System.Windows.Forms.RadioButton();
this.rbRight = new System.Windows.Forms.RadioButton();
this.rbNormal = new System.Windows.Forms.RadioButton();
this.rbButtons = new System.Windows.Forms.RadioButton();
this.rbFlatButtons = new System.Windows.Forms.RadioButton();
this.tabPage3 = new System.Windows.Forms.TabPage();
this.cbHotTrack = new System.Windows.Forms.CheckBox();
this.tabPageBorderStyle = new System.Windows.Forms.TabPage();
this.rbNone = new System.Windows.Forms.RadioButton();
this.rbFixedSingle = new System.Windows.Forms.RadioButton();
this.rbFixed3D = new System.Windows.Forms.RadioButton();
this.tabControl1.SuspendLayout();
this.tabPage1.SuspendLayout();
this.tabPage2.SuspendLayout();
this.tabPage3.SuspendLayout();
this.tabPageBorderStyle.SuspendLayout();
this.SuspendLayout();
//
// tabControl1
//
this.tabControl1.Controls.AddRange(new System.Windows.Forms.Control[] {
this.tabPage1,
this.tabPage2,
this.tabPage3,
this.tabPageBorderStyle});
this.tabControl1.Location = new System.Drawing.Point(8, 8);
this.tabControl1.Multiline = true;
this.tabControl1.Name = "tabControl1";
this.tabControl1.SelectedIndex = 0;
this.tabControl1.Size = new System.Drawing.Size(160, 152);
this.tabControl1.TabIndex = 0;
//
// tabPage1
//
this.tabPage1.Controls.AddRange(new System.Windows.Forms.Control[] {
this.rbRight,
this.rbLeft,
this.rbBottom,
this.rbTop});
this.tabPage1.Location = new System.Drawing.Point(4, 38);
this.tabPage1.Name = "tabPage1";
this.tabPage1.Size = new System.Drawing.Size(152, 110);
this.tabPage1.TabIndex = 0;
this.tabPage1.Text = "Alignment";
//
// tabPage2
//
this.tabPage2.Controls.AddRange(new System.Windows.Forms.Control[] {
this.rbFlatButtons,
this.rbButtons,
this.rbNormal});
this.tabPage2.Location = new System.Drawing.Point(4, 38);
this.tabPage2.Name = "tabPage2";
this.tabPage2.Size = new System.Drawing.Size(152, 110);
this.tabPage2.TabIndex = 1;
this.tabPage2.Text = "Appearance";
//
// rbTop
//
this.rbTop.Location = new System.Drawing.Point(16, 8);
this.rbTop.Name = "rbTop";
this.rbTop.Size = new System.Drawing.Size(96, 24);
this.rbTop.TabIndex = 0;
this.rbTop.Text = "Top";
this.rbTop.CheckedChanged += new System.EventHandler(this.rbTop_CheckedChanged);
//
// rbBottom
//
this.rbBottom.Location = new System.Drawing.Point(16, 32);
this.rbBottom.Name = "rbBottom";
this.rbBottom.Size = new System.Drawing.Size(96, 24);
this.rbBottom.TabIndex = 1;
this.rbBottom.Text = "Bottom";
this.rbBottom.CheckedChanged += new System.EventHandler(this.rbBottom_CheckedChanged);
//
// rbLeft
//
this.rbLeft.Location = new System.Drawing.Point(16, 56);
this.rbLeft.Name = "rbLeft";
this.rbLeft.Size = new System.Drawing.Size(96, 24);
this.rbLeft.TabIndex = 2;
this.rbLeft.Text = "Left";
this.rbLeft.CheckedChanged += new System.EventHandler(this.rbLeft_CheckedChanged);
//
// rbRight
//
this.rbRight.Location = new System.Drawing.Point(16, 80);
this.rbRight.Name = "rbRight";
this.rbRight.Size = new System.Drawing.Size(96, 24);
this.rbRight.TabIndex = 3;
this.rbRight.Text = "Right";
this.rbRight.CheckedChanged += new System.EventHandler(this.rbRight_CheckedChanged);
//
// rbNormal
//
this.rbNormal.Location = new System.Drawing.Point(16, 8);
this.rbNormal.Name = "rbNormal";
this.rbNormal.TabIndex = 0;
this.rbNormal.Text = "Normal";
this.rbNormal.CheckedChanged += new System.EventHandler(this.rbNormal_CheckedChanged);
//
// rbButtons
//
this.rbButtons.Location = new System.Drawing.Point(16, 32);
this.rbButtons.Name = "rbButtons";
this.rbButtons.TabIndex = 1;
this.rbButtons.Text = "Buttons";
this.rbButtons.CheckedChanged += new System.EventHandler(this.rbButtons_CheckedChanged);
//
// rbFlatButtons
//
this.rbFlatButtons.Location = new System.Drawing.Point(16, 56);
this.rbFlatButtons.Name = "rbFlatButtons";
this.rbFlatButtons.TabIndex = 2;
this.rbFlatButtons.Text = "FlatButtons";
this.rbFlatButtons.CheckedChanged += new System.EventHandler(this.rbFlatButtons_CheckedChanged);
//
// tabPage3
//
this.tabPage3.Controls.AddRange(new System.Windows.Forms.Control[] {
this.cbHotTrack});
this.tabPage3.Location = new System.Drawing.Point(4, 38);
this.tabPage3.Name = "tabPage3";
this.tabPage3.Size = new System.Drawing.Size(152, 110);
this.tabPage3.TabIndex = 2;
this.tabPage3.Text = "HotTrack";
//
// cbHotTrack
//
this.cbHotTrack.Location = new System.Drawing.Point(16, 16);
this.cbHotTrack.Name = "cbHotTrack";
this.cbHotTrack.TabIndex = 0;
this.cbHotTrack.Text = "HotTrack";
this.cbHotTrack.CheckedChanged += new System.EventHandler(this.cbHotTrack_CheckedChanged);
//
// tabPageBorderStyle
//
this.tabPageBorderStyle.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
this.tabPageBorderStyle.Controls.AddRange(new System.Windows.Forms.Control[] {
this.rbFixed3D,
this.rbFixedSingle,
this.rbNone});
this.tabPageBorderStyle.Location = new System.Drawing.Point(4, 38);
this.tabPageBorderStyle.Name = "tabPageBorderStyle";
this.tabPageBorderStyle.Size = new System.Drawing.Size(152, 110);
this.tabPageBorderStyle.TabIndex = 3;
this.tabPageBorderStyle.Text = "BorderStyle";
//
// rbNone
//
this.rbNone.Location = new System.Drawing.Point(16, 8);
this.rbNone.Name = "rbNone";
this.rbNone.TabIndex = 0;
this.rbNone.Text = "None";
this.rbNone.CheckedChanged += new System.EventHandler(this.rbNone_CheckedChanged);
//
// rbFixedSingle
//
this.rbFixedSingle.Location = new System.Drawing.Point(16, 32);
this.rbFixedSingle.Name = "rbFixedSingle";
this.rbFixedSingle.TabIndex = 1;
this.rbFixedSingle.Text = "FixedSingle";
this.rbFixedSingle.CheckedChanged += new System.EventHandler(this.rbFixedSingle_CheckedChanged);
//
// rbFixed3D
//
this.rbFixed3D.Location = new System.Drawing.Point(16, 56);
this.rbFixed3D.Name = "rbFixed3D";
this.rbFixed3D.TabIndex = 2;
this.rbFixed3D.Text = "Fixed3D";
this.rbFixed3D.CheckedChanged += new System.EventHandler(this.rbFixed3D_CheckedChanged);
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(184, 174);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.tabControl1});
this.Name = "Form1";
this.Text = "Form1";
this.tabControl1.ResumeLayout(false);
this.tabPage1.ResumeLayout(false);
this.tabPage2.ResumeLayout(false);
this.tabPage3.ResumeLayout(false);
this.tabPageBorderStyle.ResumeLayout(false);
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void rbTop_CheckedChanged(object sender, System.EventArgs e)
{
if (this.rbTop.Checked) this.tabControl1.Alignment = TabAlignment.Top;
}
private void rbLeft_CheckedChanged(object sender, System.EventArgs e)
{
if (this.rbLeft.Checked) this.tabControl1.Alignment = TabAlignment.Left;
}
private void rbRight_CheckedChanged(object sender, System.EventArgs e)
{
if (this.rbRight.Checked) this.tabControl1.Alignment = TabAlignment.Right;
}
private void rbBottom_CheckedChanged(object sender, System.EventArgs e)
{
if (this.rbBottom.Checked) this.tabControl1.Alignment = TabAlignment.Bottom;
}
private void rbNormal_CheckedChanged(object sender, System.EventArgs e)
{
if (this.rbNormal.Checked) this.tabControl1.Appearance = TabAppearance.Normal;
}
private void rbButtons_CheckedChanged(object sender, System.EventArgs e)
{
if (this.rbButtons.Checked) this.tabControl1.Appearance = TabAppearance.Buttons;
}
private void rbFlatButtons_CheckedChanged(object sender, System.EventArgs e)
{
if (this.rbFlatButtons.Checked) this.tabControl1.Appearance = TabAppearance.FlatButtons;
}
private void cbHotTrack_CheckedChanged(object sender, System.EventArgs e)
{
this.tabControl1.HotTrack = this.cbHotTrack.Enabled;
}
private void rbNone_CheckedChanged(object sender, System.EventArgs e)
{
this.tabPageBorderStyle.BorderStyle = BorderStyle.None;
}
private void rbFixedSingle_CheckedChanged(object sender, System.EventArgs e)
{
this.tabPageBorderStyle.BorderStyle = BorderStyle.FixedSingle;
}
private void rbFixed3D_CheckedChanged(object sender, System.EventArgs e)
{
this.tabPageBorderStyle.BorderStyle = BorderStyle.Fixed3D;
}
}
}