|

ツールバー
|
開発環境: Visual Studio 2003
次のような機能のForm アプリケーションを作る。
- ツールバーに画像を表示する。
- ツールバーにより、プログレスバーの値を変更する。
- 同時にステータスバーにツールバーにプログレスバーの値と、どちらのボタンが押されたかを表示する。
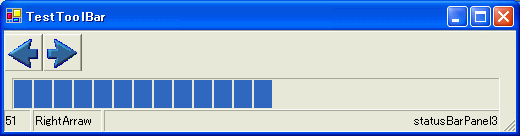
1. ファイル新規作成より、Windows アプリケーションを選択する。
2. ツールボックスより、ToolBar, ImageList, StatusBar を貼り付ける。
3, ToolBar のボタンの追加
ToolBar のプロパティのButtons コレクションの...ボタンをクリックする。(下図参照)
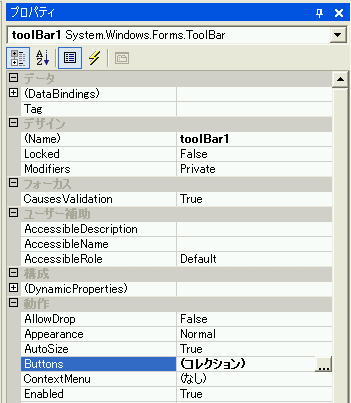
すると、次のようなImageコレクションエディタが起動する。
そこで、追加ボタンを押すことにより、自動的にボタンが追加される。ここでは、矢印用に2つのボタン登録する。(下図)
それから、どちらのボタンが押されたのか判断するために、Tag プロパティに、"Right", "Left"と入力しておく。
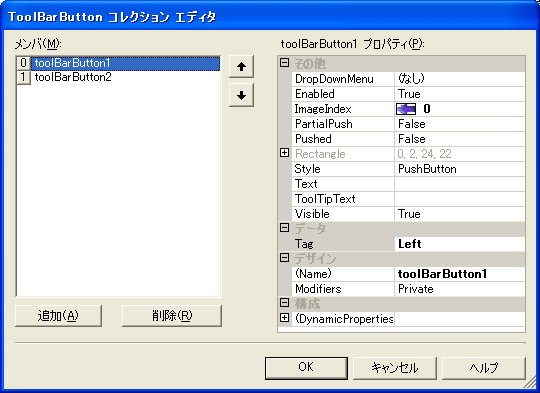
3, ToolBar にImageListを割り当てる。
このままでは、文字列のToolBar ボタンが表示されているので、ボタンに画像を貼り付ける。
このために、ToolBarのImageList プロパティをセットする。
下図のように、ToolBarのImageList プロパティは、プルダウンで選べるようになっているので、imageList1を選択する。
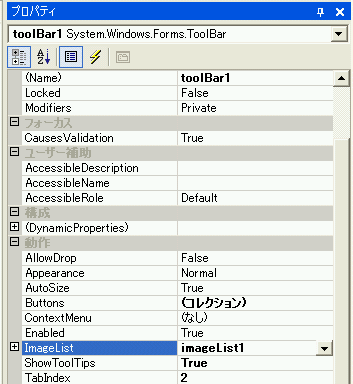
4. ImageListに画像を割り当てる。
このままでは、ImageList には画像は割り当てられてないので、画像を割り当てる。
imageList1のプロパティのImages コレクションの...ボタンをクリックし Image コレクションエディタを起動する。
ここでは、あらかじめ作っておいた矢印の画像を追加する。
追加ボタンを押すと、自動的にボタンが追加される。
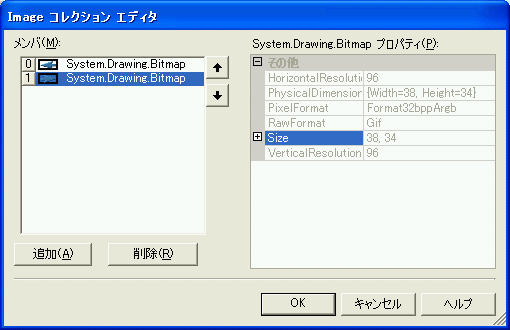
ここまでくれば、つぎのようなフォームデザインができる。
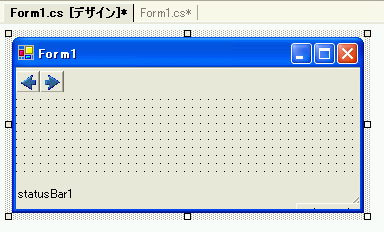
同様に、ステータスバーのプロパティのパネルコレクションから、パネルを3つ貼り付ける。
これも簡単なので、省略。
あとは、ProgressBar を貼り付けて、イベントが発生したらば ProgressBar.Value を変更すればOK.
ツールバーのどのボタンが押されたのか判定する方法 |
ToolBarのイベントは、ボタンごとではなく、ToolBar そのものに発生するので、
次のように、どのボタンが押されたのか判断して、その処理を行う必要があります。
どのツールバーのボタンが押されたのか判断するには、
ToolBar のButtonClick イベントハンドラを追加して、次のようにします。 |
(1) ボタンで判断する方法 |
// ボタンで判断する方法
if (e.Button == this.toolBarButton1)
・・・・ |
(2) ToolBarButton の Tagで判断する方法 |
// ToolBarButton の Tag に文字列をセットしておいて、それで判断する方法
if ((string)e.Button.Tag == "Right")
・・・・ |
注意 |
ProgressBar は、Minimum, Maximum を超えると, ArgumentException があがるので、
超えないようにするか、例外処理をする必要があります。
|
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace ToolBar
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.ImageList imageList1;
private System.Windows.Forms.ToolBar toolBar1;
private System.Windows.Forms.ToolBarButton toolBarButton1;
private System.Windows.Forms.ToolBarButton toolBarButton2;
private System.Windows.Forms.ProgressBar progressBar1;
private System.Windows.Forms.StatusBar statusBar1;
private System.Windows.Forms.StatusBarPanel statusBarPanel1;
private System.Windows.Forms.StatusBarPanel statusBarPanel2;
private System.Windows.Forms.StatusBarPanel statusBarPanel3;
private System.ComponentModel.IContainer components;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows フォーム デザイナで生成されたコード
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
System.Resources.ResourceManager resources = new System.Resources.ResourceManager(typeof(Form1));
this.imageList1 = new System.Windows.Forms.ImageList(this.components);
this.toolBar1 = new System.Windows.Forms.ToolBar();
this.toolBarButton1 = new System.Windows.Forms.ToolBarButton();
this.toolBarButton2 = new System.Windows.Forms.ToolBarButton();
this.progressBar1 = new System.Windows.Forms.ProgressBar();
this.statusBar1 = new System.Windows.Forms.StatusBar();
this.statusBarPanel1 = new System.Windows.Forms.StatusBarPanel();
this.statusBarPanel2 = new System.Windows.Forms.StatusBarPanel();
this.statusBarPanel3 = new System.Windows.Forms.StatusBarPanel();
((System.ComponentModel.ISupportInitialize)(this.statusBarPanel1)).BeginInit();
((System.ComponentModel.ISupportInitialize)(this.statusBarPanel2)).BeginInit();
((System.ComponentModel.ISupportInitialize)(this.statusBarPanel3)).BeginInit();
this.SuspendLayout();
//
// imageList1
//
this.imageList1.ImageSize = new System.Drawing.Size(16, 16);
this.imageList1.ImageStream = ((System.Windows.Forms.ImageListStreamer)(resources.GetObject("imageList1.ImageStream")));
this.imageList1.TransparentColor = System.Drawing.Color.Transparent;
//
// toolBar1
//
this.toolBar1.Buttons.AddRange(new System.Windows.Forms.ToolBarButton[] {
this.toolBarButton1,
this.toolBarButton2});
this.toolBar1.DropDownArrows = true;
this.toolBar1.ImageList = this.imageList1;
this.toolBar1.Location = new System.Drawing.Point(0, 0);
this.toolBar1.Name = "toolBar1";
this.toolBar1.ShowToolTips = true;
this.toolBar1.Size = new System.Drawing.Size(272, 28);
this.toolBar1.TabIndex = 0;
this.toolBar1.ButtonClick += new System.Windows.Forms.ToolBarButtonClickEventHandler(this.OnButtonClick);
//
// toolBarButton1
//
this.toolBarButton1.ImageIndex = 0;
this.toolBarButton1.Tag = "Left";
//
// toolBarButton2
//
this.toolBarButton2.ImageIndex = 1;
this.toolBarButton2.Tag = "Right";
//
// progressBar1
//
this.progressBar1.Location = new System.Drawing.Point(24, 48);
this.progressBar1.Name = "progressBar1";
this.progressBar1.Size = new System.Drawing.Size(208, 23);
this.progressBar1.TabIndex = 1;
this.progressBar1.Value = 50;
//
// statusBar1
//
this.statusBar1.Location = new System.Drawing.Point(0, 88);
this.statusBar1.Name = "statusBar1";
this.statusBar1.Panels.AddRange(new System.Windows.Forms.StatusBarPanel[] {
this.statusBarPanel1,
this.statusBarPanel2,
this.statusBarPanel3});
this.statusBar1.ShowPanels = true;
this.statusBar1.Size = new System.Drawing.Size(272, 22);
this.statusBar1.TabIndex = 2;
this.statusBar1.Text = "statusBar1";
//
// statusBarPanel1
//
this.statusBarPanel1.Text = "statusBarPanel1";
//
// statusBarPanel2
//
this.statusBarPanel2.Alignment = System.Windows.Forms.HorizontalAlignment.Center;
this.statusBarPanel2.Text = "statusBarPanel2";
//
// statusBarPanel3
//
this.statusBarPanel3.Alignment = System.Windows.Forms.HorizontalAlignment.Right;
this.statusBarPanel3.AutoSize = System.Windows.Forms.StatusBarPanelAutoSize.Spring;
this.statusBarPanel3.Text = "statusBarPanel3";
this.statusBarPanel3.Width = 56;
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(272, 110);
this.Controls.Add(this.statusBar1);
this.Controls.Add(this.progressBar1);
this.Controls.Add(this.toolBar1);
this.Name = "Form1";
this.Text = "Form1";
((System.ComponentModel.ISupportInitialize)(this.statusBarPanel1)).EndInit();
((System.ComponentModel.ISupportInitialize)(this.statusBarPanel2)).EndInit();
((System.ComponentModel.ISupportInitialize)(this.statusBarPanel3)).EndInit();
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void OnButtonClick(object sender, System.Windows.Forms.ToolBarButtonClickEventArgs e)
{
if ((string)e.Button.Tag == "Right")
{
this.statusBarPanel2.Text = "RightArrow";
if(this.progressBar1.Value >= this.progressBar1.Maximum) // Out of Range しないようにする。
goto exit; // 気持ち悪いけどこんなこともできる。
this.progressBar1.Value ++;
this.statusBarPanel1.Text = (this.progressBar1.Value).ToString();
}
else if (e.Button == this.toolBarButton1)
{
this.statusBarPanel2.Text = "LeftArrow";
try
{
this.progressBar1.Value --;
this.statusBarPanel1.Text = (this.progressBar1.Value).ToString();
}
catch (ArgumentException exc) // または、Out of Range 時の例外をキャッチする。
{
this.progressBar1.Value = 0;
this.statusBarPanel3.Text = exc.Message;
}
}
exit: // 気持ち悪いけどこんなこともできる。
return;
}
}
}