|
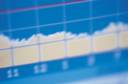
Network のパフォーマンスを表示する
|
開発環境: Visual Studio 2003
次のような機能のForm アプリケーションを作る。
- ネットワークの Total Read/Write /Sec の情報を1秒ごとに プログレスバー に表示する。
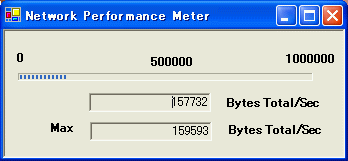
作り方
1. フォームに ツールボックスより、ProgressBar を貼り付ける。
2. フォームに ツールボックスより、タイマーを貼り付ける。
3. フォームに ツールボックスより、TextBox(現在値、Max値)を2つ貼り付ける。
4. フォームに ツールボックスより、プログレスバーのメモリ用にラベルを貼り付ける。
5. 各プロパティを次のようにセットする。
Formのプロパティ
プロパティ | 値 |
Text | Network Performance Meter |
Timerのプロパティ
プロパティ | 値 |
Enabled | True |
Interval | 1000 |
5. フォームに ツールボックスのコンポーネントより、Network Interface の Bytes
Total/Sec(任意) を貼り付ける。
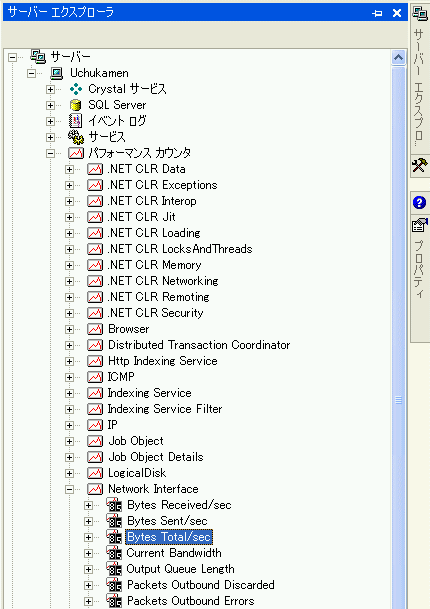
注意:
この時、InstanceName は、このコンピュータで使用されている Interface を選択する。
この場合、VIA VT86C100A-Based Fast Ethernet Adapter を選択する。
この値は、それぞれの環境で合わせないとだめ。
方法については、ヘルプ"チュートリアル : カテゴリおよびカウンタの取得"に出ていました。
簡単そうなので、やってみたらけっこうはまりました。
任意のパフォーマンスカウンターを表示するを参照してください。
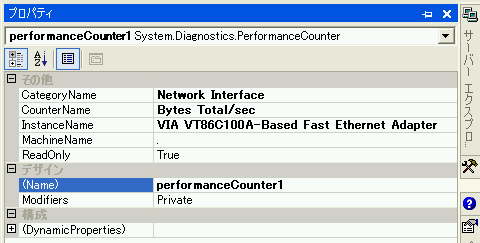
6. あとは、タイマーで1秒ごとに更新する。
このとき、プログレスバーのレンジが丁度よくなるように調整しています。
実際に書く必要があるのは、次の20数行のみ。
private int MaxValue = 0;
private void OnTick(object sender, System.EventArgs e)
{
try
{
int val = (int)this.performanceCounter1.NextValue();
while (val > 0)
{
if ( val >= this.progressBar1.Maximum)
this.progressBar1.Maximum *= 10;
else
break;
}
if (val > this.MaxValue)
{
this.MaxValue = val;
this.textBoxMax.Text = val.ToString();
}
this.labelMid.Text = (this.progressBar1.Maximum/2).ToString();
this.labelMax.Text = (this.progressBar1.Maximum).ToString();
this.progressBar1.Value = val;
this.textBox1.Text = val.ToString();
}
catch (System.ArgumentException exp)
{
this.errorProvider1.SetError(this.progressBar1, "Out of Range!" + exp.Message);
}
}
日付 | 修正履歴 |
5/5 | ちょっとした見た目を改良 |
4/29 | 初期バージョン作成 |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace PerfMeter
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.Timer timer1;
private System.Windows.Forms.ProgressBar progressBar1;
private System.Diagnostics.PerformanceCounter performanceCounter1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.ErrorProvider errorProvider1;
private System.Windows.Forms.Label labelMid;
private System.Windows.Forms.Label labelMax;
private System.Windows.Forms.TextBox textBoxMax;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label4;
private System.ComponentModel.IContainer components;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
this.performanceCounter1.BeginInit();
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
this.timer1 = new System.Windows.Forms.Timer(this.components);
this.progressBar1 = new System.Windows.Forms.ProgressBar();
this.performanceCounter1 = new System.Diagnostics.PerformanceCounter();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.textBox1 = new System.Windows.Forms.TextBox();
this.errorProvider1 = new System.Windows.Forms.ErrorProvider();
this.labelMid = new System.Windows.Forms.Label();
this.labelMax = new System.Windows.Forms.Label();
this.textBoxMax = new System.Windows.Forms.TextBox();
this.label1 = new System.Windows.Forms.Label();
this.label4 = new System.Windows.Forms.Label();
((System.ComponentModel.ISupportInitialize)(this.performanceCounter1)).BeginInit();
this.SuspendLayout();
//
// timer1
//
this.timer1.Enabled = true;
this.timer1.Interval = 1000;
this.timer1.Tick += new System.EventHandler(this.OnTick);
//
// progressBar1
//
this.progressBar1.Anchor = ((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right);
this.progressBar1.Location = new System.Drawing.Point(13, 42);
this.progressBar1.Name = "progressBar1";
this.progressBar1.Size = new System.Drawing.Size(295, 8);
this.progressBar1.Step = 1;
this.progressBar1.TabIndex = 1;
//
// performanceCounter1
//
this.performanceCounter1.CategoryName = "Network Interface";
this.performanceCounter1.CounterName = "Bytes Total/sec";
this.performanceCounter1.InstanceName = "VIA VT86C100A-Based Fast Ethernet Adapter";
//
// label2
//
this.label2.Anchor = (System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Left);
this.label2.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label2.Location = new System.Drawing.Point(219, 66);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(103, 16);
this.label2.TabIndex = 5;
this.label2.Text = "Bytes Total/Sec";
//
// label3
//
this.label3.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label3.Location = new System.Drawing.Point(10, 21);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(14, 14);
this.label3.TabIndex = 6;
this.label3.Text = "0";
//
// textBox1
//
this.textBox1.Anchor = (System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Left);
this.textBox1.Location = new System.Drawing.Point(84, 62);
this.textBox1.Name = "textBox1";
this.textBox1.ReadOnly = true;
this.textBox1.Size = new System.Drawing.Size(122, 19);
this.textBox1.TabIndex = 7;
this.textBox1.Text = "";
this.textBox1.TextAlign = System.Windows.Forms.HorizontalAlignment.Right;
//
// errorProvider1
//
this.errorProvider1.DataMember = null;
//
// labelMid
//
this.labelMid.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.labelMid.Location = new System.Drawing.Point(140, 25);
this.labelMid.Name = "labelMid";
this.labelMid.Size = new System.Drawing.Size(50, 14);
this.labelMid.TabIndex = 8;
this.labelMid.Text = "50";
this.labelMid.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// labelMax
//
this.labelMax.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.labelMax.Location = new System.Drawing.Point(270, 22);
this.labelMax.Name = "labelMax";
this.labelMax.Size = new System.Drawing.Size(67, 14);
this.labelMax.TabIndex = 9;
this.labelMax.Text = "100";
this.labelMax.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// textBoxMax
//
this.textBoxMax.Anchor = (System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Left);
this.textBoxMax.Location = new System.Drawing.Point(85, 91);
this.textBoxMax.Name = "textBoxMax";
this.textBoxMax.ReadOnly = true;
this.textBoxMax.Size = new System.Drawing.Size(122, 19);
this.textBoxMax.TabIndex = 10;
this.textBoxMax.Text = "";
this.textBoxMax.TextAlign = System.Windows.Forms.HorizontalAlignment.Right;
//
// label1
//
this.label1.Anchor = (System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Left);
this.label1.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label1.Location = new System.Drawing.Point(221, 93);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(103, 16);
this.label1.TabIndex = 11;
this.label1.Text = "Bytes Total/Sec";
//
// label4
//
this.label4.Anchor = (System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Left);
this.label4.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label4.Location = new System.Drawing.Point(43, 91);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(34, 19);
this.label4.TabIndex = 12;
this.label4.Text = "Max";
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(338, 125);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.label4,
this.label1,
this.textBoxMax,
this.labelMax,
this.labelMid,
this.textBox1,
this.label3,
this.label2,
this.progressBar1});
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.Fixed3D;
this.Name = "Form1";
this.Text = "Network Performance Meter";
((System.ComponentModel.ISupportInitialize)(this.performanceCounter1)).EndInit();
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private int MaxValue = 0;
private void OnTick(object sender, System.EventArgs e)
{
try
{
int val = (int)this.performanceCounter1.NextValue();
while (val > 0)
{
if ( val >= this.progressBar1.Maximum)
this.progressBar1.Maximum *= 10;
else
break;
}
if (val > this.MaxValue)
{
this.MaxValue = val;
this.textBoxMax.Text = val.ToString();
}
this.labelMid.Text = (this.progressBar1.Maximum/2).ToString();
this.labelMax.Text = (this.progressBar1.Maximum).ToString();
this.progressBar1.Value = val;
this.textBox1.Text = val.ToString();
}
catch (System.ArgumentException exp)
{
this.errorProvider1.SetError(this.progressBar1, "Out of Range!" + exp.Message);
}
}
}
}