|

DataGrid (その2)
|
開発環境: Visual Studio 2003次のような機能のForm アプリケーションを作る。
- データベースに接続しないで直接データソースを使う。
- 直接セルにデータを入力できる。
- 複数のテーブルをタブコントロールで表示を切り替えることができる。
- 複数のテーブルを1つのXMLファイルでセーブ、ロードができる。
Personal タブの表示例
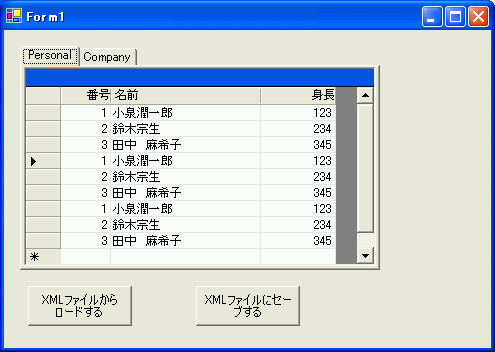
Company タブの表示例
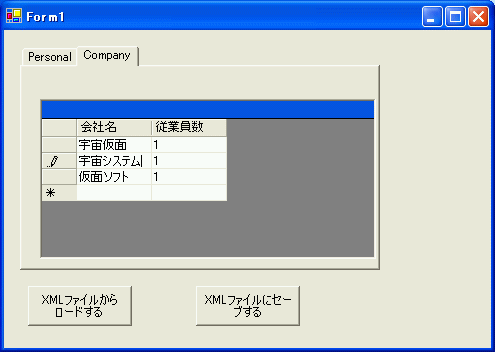
XMLファイルの表示例
1つのファイルに、PersonalInfoTable と CompanyInfoTable の両方を保存することができる。
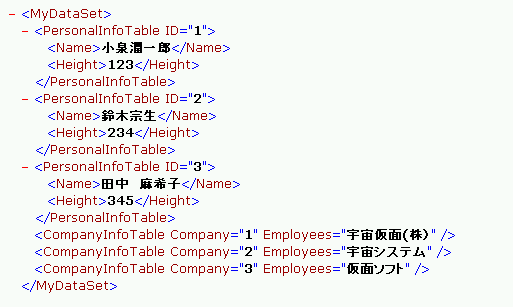
作り方
- 基本的に、DataGrid と同じ作り方で、テーブルの数とデータグリッドの数が違うだけ。
- dataSet1のプロパティから、Tables コレクションを開き、PersonalInfoTable とCompanyInfoTable
の2つのテーブルを作成する。
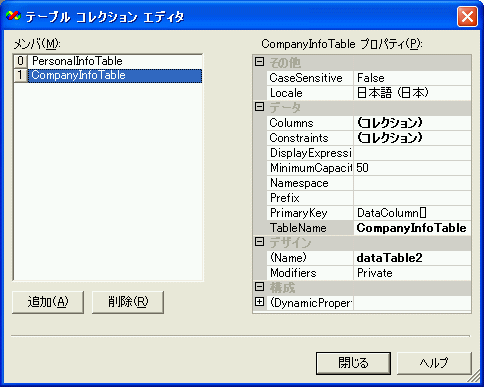
- ツールボックスより、フォームにTabControl を貼り付ける。
- タブコントロールに2つの TabSheet を追加する。
タブコントロールの TabPages コレクションの ... ボタンを押すと、次のような TabPage
コレクションエディタが表示されるので、tabPage1, tabPage2 の2つのタブページを追加する。このとき、TabPage.Text
には適当な見出し文字を入力する。(下図)
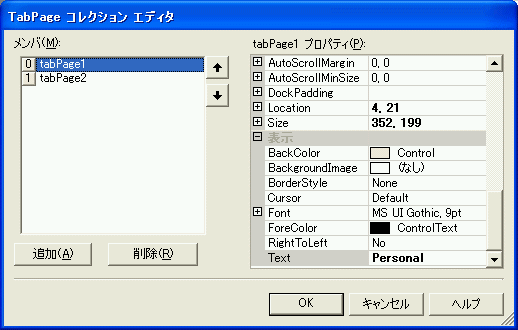
- ツールボックスより、各 TabSheet に1つづつデータグリッドをはりつける。
- データセットとデータグリッドのマッチングを取る。
dataGrid1, dataGrid2 のプロパティより、DataSource, DataMember の値を次のようにセットする。
dataGrid1 | | dataGrid2 |
Property | Value |
DataSource | dataSet1 |
DataMember | PersonalInfoTable |
| |
Property | Value |
DataSource | dataSet1 |
DataMember | CompanyInfoTable | |
- カラムスタイルを追加し、見た目を調整する。
dataGrid1のプロパティよりTableStyles コレクションの ...ボタンを押して、DataGridTableStyle
コレクション エディタを表示します。
ここで、追加ボタンを押し、dataGridTableStyle1 を追加します。(下図)
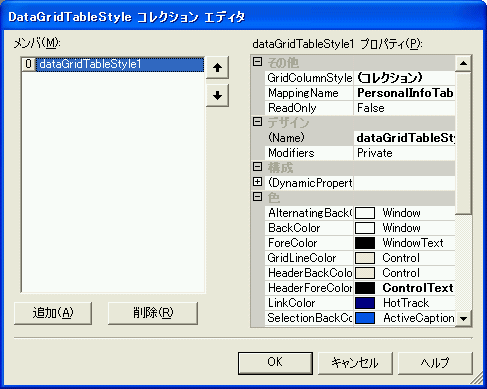
ここで、dataGridTableStyle1 のMappingName はPersonalInfoTableを選択します。
- 同様に dataGridTableStyle2 のMappingName はCompanyInfoTableを選択します。
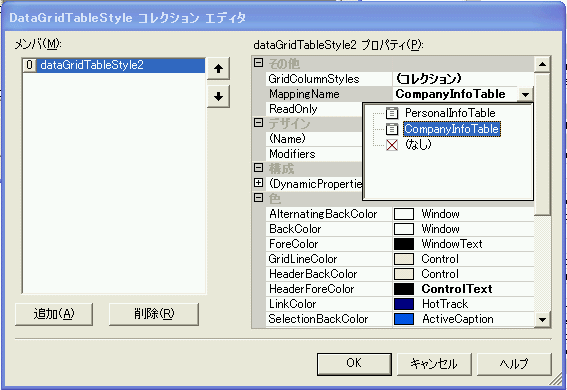
- 次に、DataGridTableStyle コレクションエディタの GridColumnStyle コレクションの
...ボタンを押して、DataGridColumnStyle コレクションエディタを開きます。ここで、各カラムのスタイルを決定します。
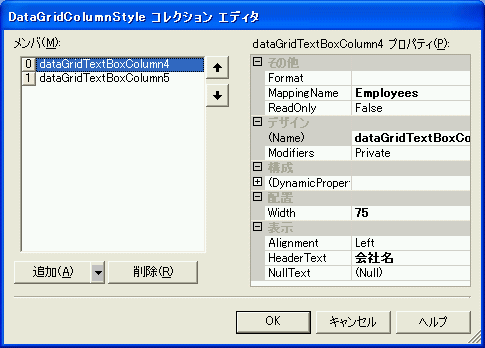
dataGridTextBoxColumn4, dataGridTextBoxColumn5 のプロパティは次のように適当にセットします。
MapppingName は直接タイプするより、コンボボックスより選択するほうが安全確実です。
dataGridTextBoxColumn4 |
Property | Value |
MappingName | Employees |
Width | 75 |
Alignment | Left |
HeaderText | 会社名 |
NullText | (Null) | |
dataGridTextBoxColumn5 |
Property | Value |
MappingName | Company |
Width | 100 |
Alignment | Left |
HeaderText | 従業員数 |
NullText | (Null) | |
- ファイルのセーブ、ロードのロジックは DataGrid1 とまったく同じでOKで、複数のテーブルを1つのファイルに入出力できます。
- テーブル間のデータのやり取りは、
this.dataTable1.Rows[1][0] = this.dataTable2.Rows[0][1];
という感じでできる。
日付 | 修正履歴 |
2002/6/27 | 初期バージョン作成 |
2002/12/06 | データセットをクリアしてから、ファイルをロードするように修正。 |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.IO;
using System.Xml;
namespace DataGrid2
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Data.DataSet dataSet1;
private System.Data.DataTable dataTable1;
private System.Data.DataColumn dataColumn1;
private System.Data.DataColumn dataColumn2;
private System.Data.DataColumn dataColumn3;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.DataGrid dataGrid1;
private System.Windows.Forms.DataGridTableStyle dataGridTableStyle1;
private System.Windows.Forms.DataGridTextBoxColumn dataGridTextBoxColumn1;
private System.Windows.Forms.DataGridTextBoxColumn dataGridTextBoxColumn2;
private System.Windows.Forms.DataGridTextBoxColumn dataGridTextBoxColumn3;
private System.Windows.Forms.TabControl tabControl1;
private System.Windows.Forms.TabPage tabPage1;
private System.Windows.Forms.TabPage tabPage2;
private System.Windows.Forms.DataGrid dataGrid2;
private System.Data.DataTable dataTable2;
private System.Data.DataColumn dataColumn4;
private System.Data.DataColumn dataColumn5;
private System.Windows.Forms.DataGridTableStyle dataGridTableStyle2;
private System.Windows.Forms.DataGridTextBoxColumn dataGridTextBoxColumn4;
private System.Windows.Forms.DataGridTextBoxColumn dataGridTextBoxColumn5;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.dataSet1 = new System.Data.DataSet();
this.dataTable1 = new System.Data.DataTable();
this.dataColumn1 = new System.Data.DataColumn();
this.dataColumn2 = new System.Data.DataColumn();
this.dataColumn3 = new System.Data.DataColumn();
this.dataTable2 = new System.Data.DataTable();
this.dataColumn4 = new System.Data.DataColumn();
this.dataColumn5 = new System.Data.DataColumn();
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.dataGrid1 = new System.Windows.Forms.DataGrid();
this.dataGridTableStyle1 = new System.Windows.Forms.DataGridTableStyle();
this.dataGridTextBoxColumn1 = new System.Windows.Forms.DataGridTextBoxColumn();
this.dataGridTextBoxColumn2 = new System.Windows.Forms.DataGridTextBoxColumn();
this.dataGridTextBoxColumn3 = new System.Windows.Forms.DataGridTextBoxColumn();
this.tabControl1 = new System.Windows.Forms.TabControl();
this.tabPage1 = new System.Windows.Forms.TabPage();
this.tabPage2 = new System.Windows.Forms.TabPage();
this.dataGrid2 = new System.Windows.Forms.DataGrid();
this.dataGridTableStyle2 = new System.Windows.Forms.DataGridTableStyle();
this.dataGridTextBoxColumn4 = new System.Windows.Forms.DataGridTextBoxColumn();
this.dataGridTextBoxColumn5 = new System.Windows.Forms.DataGridTextBoxColumn();
((System.ComponentModel.ISupportInitialize)(this.dataSet1)).BeginInit();
((System.ComponentModel.ISupportInitialize)(this.dataTable1)).BeginInit();
((System.ComponentModel.ISupportInitialize)(this.dataTable2)).BeginInit();
((System.ComponentModel.ISupportInitialize)(this.dataGrid1)).BeginInit();
this.tabControl1.SuspendLayout();
this.tabPage1.SuspendLayout();
this.tabPage2.SuspendLayout();
((System.ComponentModel.ISupportInitialize)(this.dataGrid2)).BeginInit();
this.SuspendLayout();
//
// dataSet1
//
this.dataSet1.DataSetName = "MyDataSet";
this.dataSet1.Locale = new System.Globalization.CultureInfo("ja-JP");
this.dataSet1.Tables.AddRange(new System.Data.DataTable[] {
this.dataTable1,
this.dataTable2});
//
// dataTable1
//
this.dataTable1.Columns.AddRange(new System.Data.DataColumn[] {
this.dataColumn1,
this.dataColumn2,
this.dataColumn3});
this.dataTable1.TableName = "PersonalInfoTable";
//
// dataColumn1
//
this.dataColumn1.Caption = "";
this.dataColumn1.ColumnMapping = System.Data.MappingType.Attribute;
this.dataColumn1.ColumnName = "ID";
//
// dataColumn2
//
this.dataColumn2.Caption = "";
this.dataColumn2.ColumnName = "Name";
//
// dataColumn3
//
this.dataColumn3.Caption = "";
this.dataColumn3.ColumnName = "Height";
//
// dataTable2
//
this.dataTable2.Columns.AddRange(new System.Data.DataColumn[] {
this.dataColumn4,
this.dataColumn5});
this.dataTable2.TableName = "CompanyInfoTable";
//
// dataColumn4
//
this.dataColumn4.ColumnMapping = System.Data.MappingType.Attribute;
this.dataColumn4.ColumnName = "Company";
//
// dataColumn5
//
this.dataColumn5.ColumnMapping = System.Data.MappingType.Attribute;
this.dataColumn5.ColumnName = "Employees";
//
// button1
//
this.button1.Location = new System.Drawing.Point(24, 256);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(104, 40);
this.button1.TabIndex = 1;
this.button1.Text = "XMLファイルからロードする";
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// button2
//
this.button2.Location = new System.Drawing.Point(192, 256);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(104, 40);
this.button2.TabIndex = 2;
this.button2.Text = "XMLファイルにセーブする";
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// dataGrid1
//
this.dataGrid1.DataMember = "PersonalInfoTable";
this.dataGrid1.DataSource = this.dataSet1;
this.dataGrid1.Dock = System.Windows.Forms.DockStyle.Fill;
this.dataGrid1.HeaderForeColor = System.Drawing.SystemColors.ControlText;
this.dataGrid1.Name = "dataGrid1";
this.dataGrid1.Size = new System.Drawing.Size(352, 199);
this.dataGrid1.TabIndex = 3;
this.dataGrid1.TableStyles.AddRange(new System.Windows.Forms.DataGridTableStyle[] {
this.dataGridTableStyle1});
//
// dataGridTableStyle1
//
this.dataGridTableStyle1.DataGrid = this.dataGrid1;
this.dataGridTableStyle1.GridColumnStyles.AddRange(new System.Windows.Forms.DataGridColumnStyle[] {
this.dataGridTextBoxColumn1,
this.dataGridTextBoxColumn2,
this.dataGridTextBoxColumn3});
this.dataGridTableStyle1.HeaderForeColor = System.Drawing.SystemColors.ControlText;
this.dataGridTableStyle1.MappingName = "PersonalInfoTable";
//
// dataGridTextBoxColumn1
//
this.dataGridTextBoxColumn1.Alignment = System.Windows.Forms.HorizontalAlignment.Right;
this.dataGridTextBoxColumn1.Format = "";
this.dataGridTextBoxColumn1.FormatInfo = null;
this.dataGridTextBoxColumn1.HeaderText = "番号";
this.dataGridTextBoxColumn1.MappingName = "ID";
this.dataGridTextBoxColumn1.NullText = "新規";
this.dataGridTextBoxColumn1.Width = 50;
//
// dataGridTextBoxColumn2
//
this.dataGridTextBoxColumn2.Format = "";
this.dataGridTextBoxColumn2.FormatInfo = null;
this.dataGridTextBoxColumn2.HeaderText = "名前";
this.dataGridTextBoxColumn2.MappingName = "Name";
this.dataGridTextBoxColumn2.NullText = "(入力例: 田中 麻希子)";
this.dataGridTextBoxColumn2.Width = 150;
//
// dataGridTextBoxColumn3
//
this.dataGridTextBoxColumn3.Alignment = System.Windows.Forms.HorizontalAlignment.Right;
this.dataGridTextBoxColumn3.Format = "";
this.dataGridTextBoxColumn3.FormatInfo = null;
this.dataGridTextBoxColumn3.HeaderText = "身長";
this.dataGridTextBoxColumn3.MappingName = "Height";
this.dataGridTextBoxColumn3.NullText = "(3桁以下の整数)";
this.dataGridTextBoxColumn3.Width = 75;
//
// tabControl1
//
this.tabControl1.Controls.AddRange(new System.Windows.Forms.Control[] {
this.tabPage1,
this.tabPage2});
this.tabControl1.Location = new System.Drawing.Point(16, 16);
this.tabControl1.Name = "tabControl1";
this.tabControl1.SelectedIndex = 0;
this.tabControl1.Size = new System.Drawing.Size(360, 224);
this.tabControl1.TabIndex = 4;
//
// tabPage1
//
this.tabPage1.Controls.AddRange(new System.Windows.Forms.Control[] {
this.dataGrid1});
this.tabPage1.Location = new System.Drawing.Point(4, 21);
this.tabPage1.Name = "tabPage1";
this.tabPage1.Size = new System.Drawing.Size(352, 199);
this.tabPage1.TabIndex = 0;
this.tabPage1.Text = "Personal";
//
// tabPage2
//
this.tabPage2.Controls.AddRange(new System.Windows.Forms.Control[] {
this.dataGrid2});
this.tabPage2.Location = new System.Drawing.Point(4, 21);
this.tabPage2.Name = "tabPage2";
this.tabPage2.Size = new System.Drawing.Size(352, 199);
this.tabPage2.TabIndex = 1;
this.tabPage2.Text = "Company";
//
// dataGrid2
//
this.dataGrid2.DataMember = "CompanyInfoTable";
this.dataGrid2.DataSource = this.dataSet1;
this.dataGrid2.HeaderForeColor = System.Drawing.SystemColors.ControlText;
this.dataGrid2.Location = new System.Drawing.Point(16, 32);
this.dataGrid2.Name = "dataGrid2";
this.dataGrid2.Size = new System.Drawing.Size(336, 160);
this.dataGrid2.TabIndex = 0;
this.dataGrid2.TableStyles.AddRange(new System.Windows.Forms.DataGridTableStyle[] {
this.dataGridTableStyle2});
//
// dataGridTableStyle2
//
this.dataGridTableStyle2.DataGrid = this.dataGrid2;
this.dataGridTableStyle2.GridColumnStyles.AddRange(new System.Windows.Forms.DataGridColumnStyle[] {
this.dataGridTextBoxColumn4,
this.dataGridTextBoxColumn5});
this.dataGridTableStyle2.HeaderForeColor = System.Drawing.SystemColors.ControlText;
this.dataGridTableStyle2.MappingName = "CompanyInfoTable";
//
// dataGridTextBoxColumn4
//
this.dataGridTextBoxColumn4.Format = "";
this.dataGridTextBoxColumn4.FormatInfo = null;
this.dataGridTextBoxColumn4.HeaderText = "会社名";
this.dataGridTextBoxColumn4.MappingName = "Employees";
this.dataGridTextBoxColumn4.Width = 75;
//
// dataGridTextBoxColumn5
//
this.dataGridTextBoxColumn5.Format = "";
this.dataGridTextBoxColumn5.FormatInfo = null;
this.dataGridTextBoxColumn5.HeaderText = "従業員数";
this.dataGridTextBoxColumn5.MappingName = "Company";
this.dataGridTextBoxColumn5.Width = 75;
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(392, 318);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.tabControl1,
this.button2,
this.button1});
this.Name = "Form1";
this.Text = "Form1";
((System.ComponentModel.ISupportInitialize)(this.dataSet1)).EndInit();
((System.ComponentModel.ISupportInitialize)(this.dataTable1)).EndInit();
((System.ComponentModel.ISupportInitialize)(this.dataTable2)).EndInit();
((System.ComponentModel.ISupportInitialize)(this.dataGrid1)).EndInit();
this.tabControl1.ResumeLayout(false);
this.tabPage1.ResumeLayout(false);
this.tabPage2.ResumeLayout(false);
((System.ComponentModel.ISupportInitialize)(this.dataGrid2)).EndInit();
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void button1_Click(object sender, System.EventArgs e)
{
OpenFileDialog dialog = new OpenFileDialog();
DialogResult result = dialog.ShowDialog();
if (result == DialogResult.OK)
{
// データセットをクリアする。
this.dataSet1.Clear();
// データソースに XML のデータを読み込む。
FileStream myFileStream = new FileStream(dialog.FileName, System.IO.FileMode.Open);
XmlTextReader myXmlReader = new XmlTextReader(myFileStream);
// XML ファイルから読み込む
this.dataSet1.ReadXml(myXmlReader);
myXmlReader.Close();
}
}
private void button2_Click(object sender, System.EventArgs e)
{
SaveFileDialog dialog = new SaveFileDialog();
DialogResult result = dialog.ShowDialog();
if (result == DialogResult.OK)
{
// データソースに XML のデータを書き込む。
FileStream myFileStream = new FileStream(dialog.FileName, System.IO.FileMode.Create);
XmlTextWriter myXmlWriter = new XmlTextWriter(myFileStream, System.Text.Encoding.UTF8);
// インデントをつけて書き出すように指定する。
myXmlWriter.Formatting = Formatting.Indented;
// XML ファイルに書き出す
this.dataSet1.WriteXml(myXmlWriter);
myXmlWriter.Close();
}
}
}
}