|
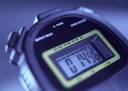
デジタルクロックを作る (その1)
|
開発環境: Visual Studio 2003
Timer, TextBox
次のような機能のForm アプリケーションを作る。
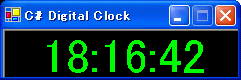
作り方 |
1. フォームに ツールボックスより、TextBox を貼り付ける。 |
2. フォームにツールボックスより、タイマーを貼り付ける。 |
3. TextBox のプロパティ、Form のプロパティのセット。 次のようにセットします。
Formのプロパティ
プロパティ | 値 |
Text | C# Digital Clock |
FormBorderStyle | FixedSingle |
TextBoxのプロパティ
プロパティ | 値 |
Font | 36ポイント(任意) |
ForeColor | Lime(任意) |
BackColor | ブラック(任意) |
TextAlign | Center |
Dock | Fill |
Cursor | No |
ReadOnly | True |
TabStop | False |
Text | 00:00:00 |
Timerのプロパティ
プロパティ | 値 |
Enabled | True |
Interval | 1000 |
|
4. ここまでくると、次のようになるはずです。
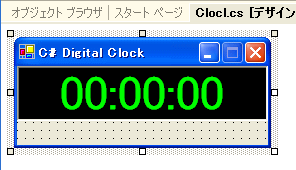
このままだと、下側が余ってしまいます。 これは、TextBox の MultiLine プロパティがデフォルト で False となっていて、なおかつ AutoSize
プロパティがデフォルトで True となっているため、TextBox が1行の高さに自動的に調整されてしまうからです。 そこで、起動時にフォームをテキストボックスのサイズに合わせるようにします。
そのために、Form のタイトルのあたりをダブルクリックします。 すると、次のように Form が始めて起動されたときに呼ばれる Load イベントが追加され、そのコードが表示されます。 この中で、this.SetClientSizeCore(this.timeTextBox.Width,
this.timeTextBox.Height); を追加します。 これは、フォームのサイズをテキストボックスのサイズに合わせるという処理です。
private void MyClock_Load(object sender, System.EventArgs e)
{
this.SetClientSizeCore(this.timeTextBox.Width, this.timeTextBox.Height);
}
|
5. このままでは、何も表示されないので、1秒ごとにタイマーで時間を表示するようにします。 Timer のプロパティより、Tick イベントに OnTick をタイプすると、自動的にイベントが追加され、コードが表示されます。 あとは、次のようにイベント発生時に、テキストボックスのTextプロパティに時間をセットすれば、表示されるようになります。
private void OnTick(object sender, System.EventArgs e) { timeTextBox.Text = DateTime.Now.ToLongTimeString(); }
|
日付 | 修正履歴 |
2002/4/28 | バックグラウンドイメージの大きさに合わせて、自動的にウィンドウをリサイズするように修正。 |
Timer コンポーネントではなく、Timer コントロールを使用するように修正。 |
2002/3/3 | 初期バージョン作成 |
2003/12/2 | Visual Studio 2003 対応版 |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace MyClock
{
/// <summary>
/// MyClockです。
/// </summary>
public class MyClock : System.Windows.Forms.Form
{
private System.Windows.Forms.Timer timer1;
private System.Windows.Forms.TextBox textBox1;
private System.ComponentModel.IContainer components;
public MyClock()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要です。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
System.Resources.ResourceManager resources = new System.Resources.ResourceManager(typeof(MyClock));
this.timer1 = new System.Windows.Forms.Timer(this.components);
this.textBox1 = new System.Windows.Forms.TextBox();
this.SuspendLayout();
//
// timer1
//
this.timer1.Enabled = true;
this.timer1.Interval = 1000;
this.timer1.Tick += new System.EventHandler(this.OnTick);
//
// textBox1
//
this.textBox1.BackColor = System.Drawing.Color.Black;
this.textBox1.Font = new System.Drawing.Font("MS UI Gothic", 24F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.textBox1.ForeColor = System.Drawing.Color.Lime;
this.textBox1.Location = new System.Drawing.Point(7, 13);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(164, 39);
this.textBox1.TabIndex = 1;
this.textBox1.Text = "";
this.textBox1.TextAlign = System.Windows.Forms.HorizontalAlignment.Center;
//
// MyClock
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.BackgroundImage = ((System.Drawing.Image)(resources.GetObject("$this.BackgroundImage")));
this.ClientSize = new System.Drawing.Size(388, 286);
this.Controls.Add(this.textBox1);
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedSingle;
this.MaximizeBox = false;
this.Name = "MyClock";
this.Text = "C# Digital Clock";
this.Load += new System.EventHandler(this.MyClock_Load);
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new MyClock());
}
private void OnTick(object sender, System.EventArgs e)
{
this.textBox1.Text = DateTime.Now.ToLongTimeString();
}
private void FitToBackgroundImage()
{
this.SetClientSizeCore(this.BackgroundImage.Width, this.BackgroundImage.Height);
}
private void MyClock_Load(object sender, System.EventArgs e)
{
this.FitToBackgroundImage();
}
}
}