|

OpenFileDialog / SaveFileDialog
|
開発環境: Visual Studio 2003
目的
|
ファイルダイアログは結構使うことがあるので、テンプレートとして一応メモっておきます。
次のような機能のForm アプリケーションを作る。
- SJIS テキストファイルから読み、TextBox に表示する。
- TextBox の内容を SJIS テキストファイルとして書き出す。
- ファイルの指定には、OpenFileDialog/SaveFileDialog を使う。
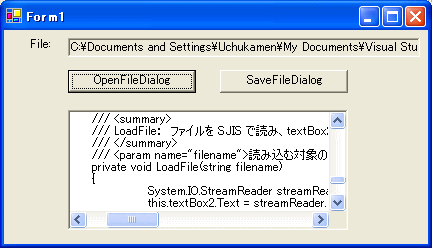
|
OpenFileDialog の処理 |
/// <summary>
/// OpenFileDialog の処理
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button1_Click(object sender, System.EventArgs e)
{
this.textBox1.Text = "";
openFileDialog1.InitialDirectory = @"..\..\" ;
openFileDialog1.Filter = "C# files (*.cs)|*.cs|Resource files (*.resx)|*.resx|Solution File (*.sln)|*.sln|All files (*.*)|*.*" ;
openFileDialog1.FilterIndex = 1 ;
openFileDialog1.RestoreDirectory = true ;
if(openFileDialog1.ShowDialog() == DialogResult.OK)
{
this.textBox1.Text = openFileDialog1.FileName;
}
}
|
SaveFileDialog の処理
|
/// <summary>
/// SaveFileDialog の処理
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button2_Click(object sender, System.EventArgs e)
{
this.textBox1.Text = "";
saveFileDialog1.InitialDirectory = @"..\..\" ;
saveFileDialog1.Filter = "C# files (*.cs)|*.cs|Resource files (*.resx)|*.resx|Solution File (*.sln)|*.sln|All files (*.*)|*.*" ;
saveFileDialog1.FilterIndex = 1 ;
saveFileDialog1.RestoreDirectory = true ;
if(saveFileDialog1.ShowDialog() == DialogResult.OK)
{
this.textBox1.Text = saveFileDialog1.FileName;
}
}
|
LoadFileDialog の処理
|
テキストファイルの読み込みには、ラインごとの読み込みができる StreamReader が便利。 一度、ファイルストリームを作って、そのファイルストリームを作るやり方もできるが、直接 StreamReader
を生成できるので、ファイルからテキストファイルを読み込むのは、次の3行でOK。 注意: この例では、例外は拾ってないので、適当に処理してください。
System.IO.StreamReader streamReader = new System.IO.StreamReader(filename, System.Text.Encoding.GetEncoding("Shift_JIS"));
this.textBox2.Text = streamReader.ReadToEnd();
streamReader.Close();
|
SaveFileDialog の処理
|
テキストファイルの書き出しには、StreamWriter が便利。 一度、ファイルストリームを作って、そのファイルストリームを作るやり方もできるが、直接 StreamWriter
を生成できるので、テキストボックスを書き出すには、次の3行でOK。 注意: この例では、例外は拾ってないので、適当に処理してください。
System.IO.StreamWriter streamWriter = new System.IO.StreamWriter(filename, false, System.Text.Encoding.GetEncoding("Shift_JIS"));
streamWriter.Write(this.textBox2.Text);
streamWriter.Close();
|
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace FileDialog
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.OpenFileDialog openFileDialog1;
private System.Windows.Forms.SaveFileDialog saveFileDialog1;
private System.Windows.Forms.TextBox textBox2;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.label1 = new System.Windows.Forms.Label();
this.textBox1 = new System.Windows.Forms.TextBox();
this.openFileDialog1 = new System.Windows.Forms.OpenFileDialog();
this.saveFileDialog1 = new System.Windows.Forms.SaveFileDialog();
this.textBox2 = new System.Windows.Forms.TextBox();
this.SuspendLayout();
//
// button1
//
this.button1.Location = new System.Drawing.Point(64, 40);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(128, 23);
this.button1.TabIndex = 0;
this.button1.Text = "OpenFileDialog";
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// button2
//
this.button2.Location = new System.Drawing.Point(216, 40);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(128, 23);
this.button2.TabIndex = 1;
this.button2.Text = "SaveFileDialog";
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// label1
//
this.label1.Location = new System.Drawing.Point(24, 8);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(32, 16);
this.label1.TabIndex = 2;
this.label1.Text = "File:";
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(64, 8);
this.textBox1.Name = "textBox1";
this.textBox1.ReadOnly = true;
this.textBox1.ScrollBars = System.Windows.Forms.ScrollBars.Horizontal;
this.textBox1.Size = new System.Drawing.Size(352, 19);
this.textBox1.TabIndex = 3;
this.textBox1.Text = "textBox1";
//
// textBox2
//
this.textBox2.Location = new System.Drawing.Point(64, 80);
this.textBox2.Multiline = true;
this.textBox2.Name = "textBox2";
this.textBox2.ScrollBars = System.Windows.Forms.ScrollBars.Both;
this.textBox2.Size = new System.Drawing.Size(280, 120);
this.textBox2.TabIndex = 4;
this.textBox2.Text = "textBox2";
this.textBox2.WordWrap = false;
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(424, 214);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.textBox2,
this.textBox1,
this.label1,
this.button2,
this.button1});
this.Name = "Form1";
this.Text = "Form1";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
/// <summary>
/// OpenFileDialog の処理
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button1_Click(object sender, System.EventArgs e)
{
this.textBox1.Text = "";
openFileDialog1.InitialDirectory = @"..\..\" ;
openFileDialog1.Filter = "C# files (*.cs)|*.cs|Resource files (*.resx)|*.resx|Solution File (*.sln)|*.sln|All files (*.*)|*.*" ;
openFileDialog1.FilterIndex = 1 ;
openFileDialog1.RestoreDirectory = true ;
if(openFileDialog1.ShowDialog() == DialogResult.OK)
{
this.textBox1.Text = openFileDialog1.FileName;
this.LoadFile(openFileDialog1.FileName);
}
}
/// <summary>
/// LoadFile: ファイルを SJIS で読み、textBox2 へ書き出す。
/// </summary>
/// <param name="filename">読み込む対象のファイルパス</param>
private void LoadFile(string filename)
{
System.IO.StreamReader streamReader = new System.IO.StreamReader(filename, System.Text.Encoding.GetEncoding("Shift_JIS"));
this.textBox2.Text = streamReader.ReadToEnd();
streamReader.Close();
}
/// <summary>
/// SaveFileDialog の処理
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button2_Click(object sender, System.EventArgs e)
{
this.textBox1.Text = "";
saveFileDialog1.InitialDirectory = @"..\..\" ;
saveFileDialog1.Filter = "C# files (*.cs)|*.cs|Resource files (*.resx)|*.resx|Solution File (*.sln)|*.sln|All files (*.*)|*.*" ;
saveFileDialog1.FilterIndex = 1 ;
saveFileDialog1.RestoreDirectory = true ;
if(saveFileDialog1.ShowDialog() == DialogResult.OK)
{
this.textBox1.Text = saveFileDialog1.FileName;
this.SaveFile(saveFileDialog1.FileName);
}
}
private void SaveFile(string filename)
{
System.IO.StreamWriter streamWriter = new System.IO.StreamWriter(filename, false, System.Text.Encoding.GetEncoding("Shift_JIS"));
streamWriter.Write(this.textBox2.Text);
streamWriter.Close();
}
}
}
2003/1/28 sjis を Shift_JIS へ訂正。