|
TextBox, RichTextBox のカットアンドペースト機能をメニューからも使えるようにする。
|
|
- 目的
- 目次
- 参考
- メインメニューの作り方
- メニューにショートカットキーを追加する。
- メニュー項目にアクセス キーを追加する。
- メニュー項目を無効にする。
- メニュー項目を一時的に表示しない。
- メニュー項目にチェックマークをつける。
- メニュー項目にラジオチェックマークをつける。
- メニューにカットアンドペーストを追加する。
- メニューアイテムをマージして、新しいメニューを作る。
- サンプルコード
|
|
(1)Windows.Forms
メニュー拡張機能 ( VS.Net のヘルプ ) (2)カットアンドペースト
( VS.NET のヘルプ )
|
|
1. ツールボックスから、メインメニューをWindows.Forms 上にドラッグアンドドロップします。 2. メインメニューが表示されるので、各メニューアイテムをクリックし、メニューの文字列を記入します。 3. 必要に応じて、ショートカットキー、アクセスキーを追加します。
|
|
メニューアイテムのプロパティから設定できます。 メニューアイテムをマウスで右クリックし、プロパティを表示します。(下図) ここで、Shortcut プロパティのドロップダウンリストを押すと、ダ〜〜ッと選択肢が現れますので、適切なものを選びます。
プログラムでは、 myMnuItem.Shortcut = System.Windows.Forms.Shortcut.CtrlC;
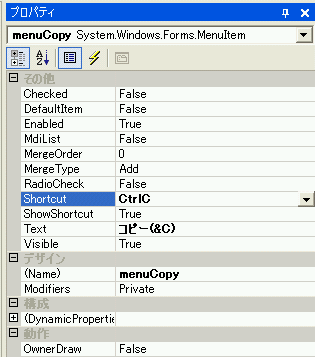
また、ShowShortcut プロパティを True にすると、メニュー上に自動的にショートカットキーが表示されます(下図)。
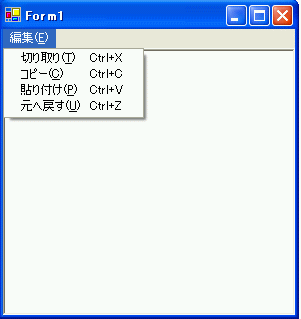
|
|
アクセスキーとは、メニュで”コピー(C)” というように、アンダーバーCが表示され、このキーを押すことにより、キーボードから選択できるようにするためのものです。 アクセスキーも、メニューアイテムのプロパティから設定できます。 メニューアイテムをマウスで右クリックし、プロパティを表示します。(上図) ここで、Text プロパティで、&C というように、&と次の1文字がアクセスキーとして設定されます。
|
|
メニューアイテムの Enabled プロパティを False にします(下図)。
プログラムでは、 myMnuItem.Enabled = false;
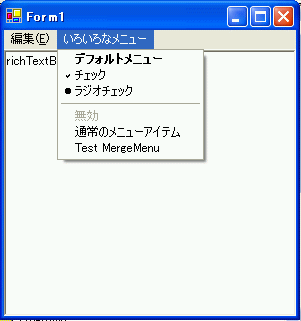
|
|
メニューアイテムの Visible プロパティを False にします。
プログラムでは、 myMnuItem.Visible = false;
|
|
メニューアイテムの Checked プロパティを True にします。 メニューアイテムの RadioChecked プロパティを False にします。
プログラムでは、 myMnuItem.RadioChecked = false; myMnuItem.Checked = true;
|
|
メニューアイテムの Checked プロパティを True にします。 メニューアイテムの RadioChecked プロパティを True にします。
プログラムでは、 myMnuItem.RadioChecked = true; myMnuItem.Checked = true;
|
|
TextBox, RichTextBox は、TextBoxBase クラスから継承しています。 TextBoxBase クラスでは、カットアンドペーストのための Cut(), Copy(), Paste(), Undo()
が用意されているので、これらをメニューから呼び出すようにしてあげればOKです。 通常は、TextBoxBase クラスから継承して新しいクラスを作ることはせず、TextBox, RichTextBox から継承します。
Cut |
private void menuCut_Click(object sender, System.EventArgs e)
{
if(this.richTextBox1.SelectionLength > 0)
this.richTextBox1.Cut();
}
|
Copy |
private void menuCopy_Click(object sender, System.EventArgs e)
{
if(this.richTextBox1.SelectionLength > 0)
this.richTextBox1.Copy();
}
|
Paste |
private void menuPaste_Click(object sender, System.EventArgs e)
{
if(Clipboard.GetDataObject().GetDataPresent(DataFormats.Text) == true)
{
if(this.richTextBox1.SelectionLength > 0)
{
this.richTextBox1.SelectionStart = this.richTextBox1.SelectionStart + this.richTextBox1.SelectionLength;
}
this.ric
hTextBox1.Paste();
}
}
|
Undo |
private void menuUndo_Click(object sender, System.EventArgs e)
{
if(this.richTextBox1.CanUndo == true)
{
this.richTextBox1.Undo();
this.richTextBox1.ClearUndo();
}
}
|
|
|
すでにあるメニューアイテムをマージして、新しいメニューを作ることができます。 たとえば、メインメニューで作成したメニューのうち、いくつかの項目だけ、コンテキストメニューに表示したい場合があると思います。 そのような場合に便利ですね。
ここでは、編集メニューといろいろなメニューをマージしたものを、コンテキストメニューとして表示しています(下図)。
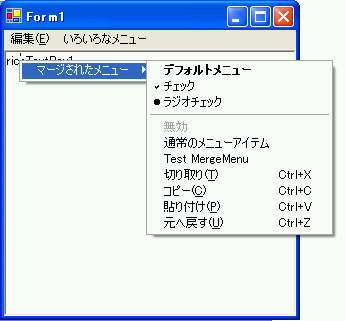
作り方 1. メインメニューを作る。 2. ツールボックスよりコンテキストメニューをドラッグアンドドロップする。 3. 適当なメニュー(ここでは、Test MergeMenu) を追加して、イベントハンドらを追加する。 4. イベントハンドらに、次のような処理を書く。
private void menuTestMergeMenu_Click(object sender, System.EventArgs e)
{
// メニューの追加方法を指定
mainMenuEdit.MergeType = MenuMerge.Add;
mainMenuMisc.MergeType = MenuMerge.MergeItems;
// メニューのコピーを作る。
MenuItem tempMenuItem = mainMenuMisc.CloneMenu();
// 編集メニューをマージする。
tempMenuItem.MergeMenu( mainMenuEdit.CloneMenu());
// 新しいメニューアイテムのタイトルを決める。
tempMenuItem.Text = "マージされたメニュー";
// コンテキストメニューに追加する。
this.contextMenu1.MenuItems.Add(tempMenuItem);
}
|
|
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Command
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.RichTextBox richTextBox1;
private System.Windows.Forms.MainMenu mainMenu1;
private System.Windows.Forms.MenuItem mainMenuEdit;
private System.Windows.Forms.MenuItem menuCut;
private System.Windows.Forms.MenuItem menuCopy;
private System.Windows.Forms.MenuItem menuPaste;
private System.Windows.Forms.MenuItem menuUndo;
private System.Windows.Forms.MenuItem menuItem6;
private System.Windows.Forms.MenuItem mainMenuMisc;
private System.Windows.Forms.MenuItem menuItemDefault;
private System.Windows.Forms.MenuItem menuCheck;
private System.Windows.Forms.MenuItem menuRadioCheck;
private System.Windows.Forms.MenuItem menuEnabled;
private System.Windows.Forms.MenuItem menuItemNormal;
private System.Windows.Forms.MenuItem menuTestMergeMenu;
private System.Windows.Forms.ContextMenu contextMenu1;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.richTextBox1 = new System.Windows.Forms.RichTextBox();
this.mainMenu1 = new System.Windows.Forms.MainMenu();
this.mainMenuEdit = new System.Windows.Forms.MenuItem();
this.menuCut = new System.Windows.Forms.MenuItem();
this.menuCopy = new System.Windows.Forms.MenuItem();
this.menuPaste = new System.Windows.Forms.MenuItem();
this.menuUndo = new System.Windows.Forms.MenuItem();
this.mainMenuMisc = new System.Windows.Forms.MenuItem();
this.menuItemDefault = new System.Windows.Forms.MenuItem();
this.menuCheck = new System.Windows.Forms.MenuItem();
this.menuRadioCheck = new System.Windows.Forms.MenuItem();
this.menuEnabled = new System.Windows.Forms.MenuItem();
this.menuItemNormal = new System.Windows.Forms.MenuItem();
this.menuItem6 = new System.Windows.Forms.MenuItem();
this.menuTestMergeMenu = new System.Windows.Forms.MenuItem();
this.contextMenu1 = new System.Windows.Forms.ContextMenu();
this.SuspendLayout();
//
// richTextBox1
//
this.richTextBox1.ContextMenu = this.contextMenu1;
this.richTextBox1.Dock = System.Windows.Forms.DockStyle.Fill;
this.richTextBox1.Name = "richTextBox1";
this.richTextBox1.Size = new System.Drawing.Size(292, 266);
this.richTextBox1.TabIndex = 0;
this.richTextBox1.Text = "richTextBox1";
//
// mainMenu1
//
this.mainMenu1.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.mainMenuEdit, this.mainMenuMisc});
//
// mainMenuEdit
//
this.mainMenuEdit.Index = 0;
this.mainMenuEdit.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.menuCut, this.menuCopy, this.menuPaste, this.menuUndo});
this.mainMenuEdit.Text = "編集(&E)";
//
// menuCut
//
this.menuCut.Index = 0;
this.menuCut.Shortcut = System.Windows.Forms.Shortcut.CtrlX;
this.menuCut.Text = "切り取り(&T)";
this.menuCut.Click += new System.EventHandler(this.menuCut_Click);
//
// menuCopy
//
this.menuCopy.Index = 1;
this.menuCopy.Shortcut = System.Windows.Forms.Shortcut.CtrlC;
this.menuCopy.Text = "コピー(&C)";
this.menuCopy.Click += new System.EventHandler(this.menuCopy_Click);
//
// menuPaste
//
this.menuPaste.Index = 2;
this.menuPaste.Shortcut = System.Windows.Forms.Shortcut.CtrlV;
this.menuPaste.Text = "貼り付け(&P)";
this.menuPaste.Click += new System.EventHandler(this.menuPaste_Click);
//
// menuUndo
//
this.menuUndo.Index = 3;
this.menuUndo.Shortcut = System.Windows.Forms.Shortcut.CtrlZ;
this.menuUndo.Text = "元へ戻す(&U)";
this.menuUndo.Click += new System.EventHandler(this.menuUndo_Click);
//
// mainMenuMisc
//
this.mainMenuMisc.Index = 1;
this.mainMenuMisc.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.menuItemDefault, this.menuCheck, this.menuRadioCheck,
this.menuItem6, this.menuEnabled,this.menuItemNormal,this.menuTestMergeMenu});
this.mainMenuMisc.Text = "いろいろなメニュー";
//
// menuItemDefault
//
this.menuItemDefault.DefaultItem = true;
this.menuItemDefault.Index = 0;
this.menuItemDefault.Text = "デフォルトメニュー";
//
// menuCheck
//
this.menuCheck.Checked = true;
this.menuCheck.Index = 1;
this.menuCheck.Text = "チェック";
//
// menuRadioCheck
//
this.menuRadioCheck.Checked = true;
this.menuRadioCheck.Index = 2;
this.menuRadioCheck.MergeType = System.Windows.Forms.MenuMerge.Replace;
this.menuRadioCheck.RadioCheck = true;
this.menuRadioCheck.Text = "ラジオチェック";
//
// menuEnabled
//
this.menuEnabled.Enabled = false;
this.menuEnabled.Index = 4;
this.menuEnabled.Text = "無効";
//
// menuItemNormal
//
this.menuItemNormal.Index = 5;
this.menuItemNormal.Text = "通常のメニューアイテム";
//
// menuItem6
//
this.menuItem6.Index = 3;
this.menuItem6.Text = "-";
//
// menuTestMergeMenu
//
this.menuTestMergeMenu.Index = 6;
this.menuTestMergeMenu.Text = "Test MergeMenu";
this.menuTestMergeMenu.Click +=
new System.EventHandler(this.menuTestMergeMenu_Click);
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(292, 266);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.richTextBox1});
this.Menu = this.mainMenu1;
this.Name = "Form1";
this.Text = "Form1";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void menuCut_Click(object sender, System.EventArgs e)
{
if(this.richTextBox1.SelectionLength > 0)
this.richTextBox1.Cut();
}
private void menuCopy_Click(object sender, System.EventArgs e)
{
if(this.richTextBox1.SelectionLength > 0)
this.richTextBox1.Copy();
}
private void menuPaste_Click(object sender, System.EventArgs e)
{
if(Clipboard.GetDataObject().GetDataPresent(DataFormats.Text) == true)
{
if(this.richTextBox1.SelectionLength > 0)
{
this.richTextBox1.SelectionStart =
this.richTextBox1.SelectionStart + this.richTextBox1.SelectionLength;
}
this.richTextBox1.Paste();
}
}
private void menuUndo_Click(object sender, System.EventArgs e)
{
if(this.richTextBox1.CanUndo == true)
{
this.richTextBox1.Undo();
this.richTextBox1.ClearUndo();
}
}
private void menuTestMergeMenu_Click(object sender, System.EventArgs e)
{
// メニューの追加方法を指定
mainMenuEdit.MergeType = MenuMerge.Add;
mainMenuMisc.MergeType = MenuMerge.MergeItems;
// メニューのコピーを作る。
MenuItem tempMenuItem = mainMenuMisc.CloneMenu();
// 編集メニューをマージする。
tempMenuItem.MergeMenu( mainMenuEdit.CloneMenu());
// 新しいメニューアイテムのタイトルを決める。
tempMenuItem.Text = "マージされたメニュー";
// コンテキストメニューに追加する。
this.contextMenu1.MenuItems.Add(tempMenuItem);
}
}
}
|