|

タスクトレイにアイコンを表示する (NotifyIcon)
|
開発環境: Visual Studio 2003
次のような機能のアプリケーションを作る。
・タスクトレイにアイコンを表示する。
・タスクトレイのアイコンを右クリックすると、メニューが表示される。
・メニューは、Menu1(ダイアログを表示), Exit(終了)。
作り方
1. Windows Forms の新規プロジェクトを TaskTray(任意) という名前で作る。
2. ツールボックスより、NotifyIcon コントロールを貼り付ける。
3. ツールボックスより、ContextMenu コントロールを貼り付ける。
4. ソリューション エクスプローラーより、TaskTray を選択し、
マウス右ボタン→追加→新しい項目の追加を選択する。
すると、次のようなダイアログが表示されるので、アイコン ファイルを選択して開くボタンを押す。
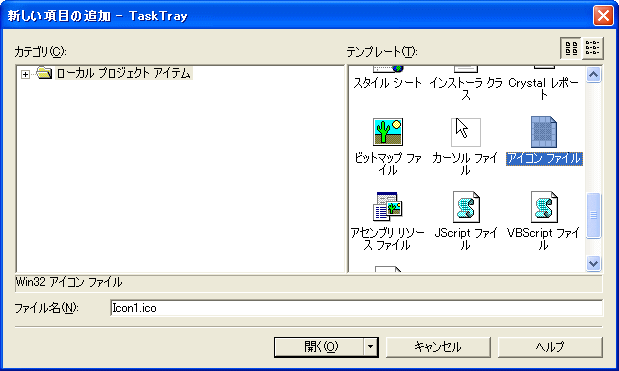
5. アイコンエディタで適当な絵を書く。
6. 2で作成したNotifyIconのプロパティシートを開き、ContextMenu に 3で作成した
contextMenu1 を登録する。
7. 次に、NotifyIconのプロパティシートの Iconに、 5 で作ったアイコンファイルを登録する。
次の図参照。
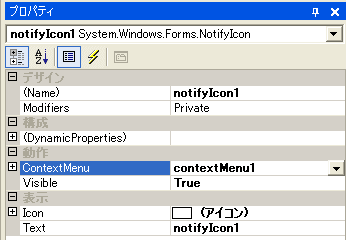
8. 3で作成したContextMenu に、フォームデザインで適当なメニューを作る。
9. 8 で作成したコンテクストメニューが選択された場合のイベント処理を、
メニュープロパティのイベントで設定する。次の図参照。
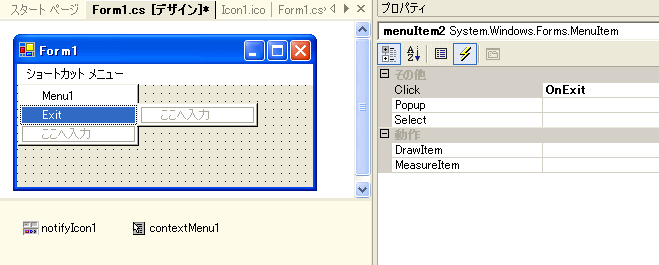
10. 9 で追加したしたイベント処理を追加する。この例ではメッセージボックスを表示する。
private void OnMenu1(object sender, System.EventArgs e)
{
MessageBox.Show("Menu1", this.Text, MessageBoxButtons.OK,
MessageBoxIcon.Exclamation);
}
private void OnExit(object sender, System.EventArgs e)
{
Application.Exit();
}
11. このままだとアプリケーションのウィンドウが表示されるので、
必要に応じてフォームウィンドウを非表示にする。このためには、Form のプロパティより、
WindowsState を Minimized、ShowInTaskBar を False に設定する。
12. 以上で、次のようなタスクトレイ上にアイコンとメニューを出すことができる。
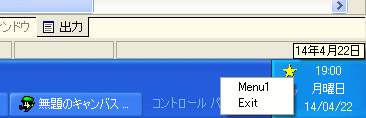
ソースコードは次のとおり。
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace TaskTray
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.NotifyIcon notifyIcon1;
private System.Windows.Forms.ContextMenu contextMenu1;
private System.Windows.Forms.MenuItem menuItem1;
private System.Windows.Forms.MenuItem menuItem2;
private System.ComponentModel.IContainer components;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に
// コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
System.Resources.ResourceManager resources =
new System.Resources.ResourceManager(typeof(Form1));
this.notifyIcon1 = new System.Windows.Forms.NotifyIcon(
this.components);
this.contextMenu1 = new System.Windows.Forms.ContextMenu();
this.menuItem1 = new System.Windows.Forms.MenuItem();
this.menuItem2 = new System.Windows.Forms.MenuItem();
//
// notifyIcon1
//
this.notifyIcon1.ContextMenu = this.contextMenu1;
this.notifyIcon1.Icon = ((System.Drawing.Icon)
(resources.GetObject("notifyIcon1.Icon")));
this.notifyIcon1.Text = "notifyIcon1";
this.notifyIcon1.Visible = true;
//
// contextMenu1
//
this.contextMenu1.MenuItems.AddRange(
new System.Windows.Forms.MenuItem[] {
this.menuItem1,
this.menuItem2});
//
// menuItem1
//
this.menuItem1.Index = 0;
this.menuItem1.Text = "Menu1";
this.menuItem1.Click += new System.EventHandler(this.OnMenu1);
//
// menuItem2
//
this.menuItem2.Index = 1;
this.menuItem2.Text = "Exit";
this.menuItem2.Click += new System.EventHandler(this.OnExit);
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(296, 102);
this.Name = "Form1";
this.ShowInTaskbar = false;
this.Text = "Form1";
this.WindowState = System.Windows.Forms.FormWindowState.
Minimized;
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void OnMenu1(object sender, System.EventArgs e)
{
MessageBox.Show("Menu1", this.Text, MessageBoxButtons.OK,
MessageBoxIcon.Exclamation);
}
private void OnExit(object sender, System.EventArgs e)
{
Application.Exit();
}
}
}