|

DNS 名前解決
|
開発環境: Visual Studio 2003
次のような機能のForm アプリケーションを作る。
- ホスト名から IP Address に変換し、ComboBox に表示する。
- IP Addressから ホスト名に変換する。
- 変換できずにタイムアウトが発生する可能性があるので、変換時には ウェイトカーソルにする。
- ホスト名、IPAdress のフォーマットが不正な場合には、ダイアログを表示す。
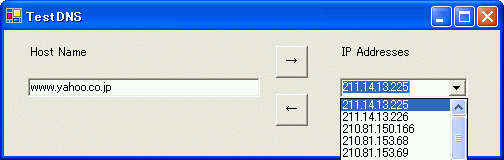
この例では、www.yahoo.co.jp に複数のIP アドレスが割り振られているのがわかる。
実行形式とソースコード一式のダウンロード 注意: テスト用です。ダウンロードしないでください。
ソースコードはコメントを参照。
以下ソースコード。
2002/4/28 OnByName メソッドで例外発生時に ComboBox
の描画を再開できないバグを修正。
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Net;
namespace TestDNS
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class TestDNS : System.Windows.Forms.Form
{
private System.Windows.Forms.Button ButtonByAddress;
private System.Windows.Forms.Button ButtonByName;
private System.Windows.Forms.TextBox hostName;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.ComboBox combIPAddress;
private System.Windows.Forms.Label label2;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public TestDNS()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.ButtonByAddress = new System.Windows.Forms.Button();
this.ButtonByName = new System.Windows.Forms.Button();
this.hostName = new System.Windows.Forms.TextBox();
this.label1 = new System.Windows.Forms.Label();
this.combIPAddress = new System.Windows.Forms.ComboBox();
this.label2 = new System.Windows.Forms.Label();
this.SuspendLayout();
//
// ButtonByAddress
//
this.ButtonByAddress.Location = new System.Drawing.Point(272, 64);
this.ButtonByAddress.Name = "ButtonByAddress";
this.ButtonByAddress.Size = new System.Drawing.Size(32, 32);
this.ButtonByAddress.TabIndex = 1;
this.ButtonByAddress.Text = "←";
this.ButtonByAddress.MouseDown += new System.Windows.Forms.MouseEventHandler(this.OnByAddress);
//
// ButtonByName
//
this.ButtonByName.Location = new System.Drawing.Point(272, 16);
this.ButtonByName.Name = "ButtonByName";
this.ButtonByName.Size = new System.Drawing.Size(32, 32);
this.ButtonByName.TabIndex = 2;
this.ButtonByName.Text = "→";
this.ButtonByName.MouseDown += new System.Windows.Forms.MouseEventHandler(this.OnByName);
//
// hostName
//
this.hostName.Location = new System.Drawing.Point(24, 48);
this.hostName.Name = "hostName";
this.hostName.Size = new System.Drawing.Size(232, 19);
this.hostName.TabIndex = 3;
this.hostName.Text = "Hostname";
//
// label1
//
this.label1.Location = new System.Drawing.Point(336, 16);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(128, 16);
this.label1.TabIndex = 5;
this.label1.Text = "IP Addresses";
//
// combIPAddress
//
this.combIPAddress.Location = new System.Drawing.Point(336, 48);
this.combIPAddress.Name = "combIPAddress";
this.combIPAddress.Size = new System.Drawing.Size(128, 20);
this.combIPAddress.TabIndex = 6;
this.combIPAddress.Text = "xxx.xxx.xxx.xxx";
//
// label2
//
this.label2.Location = new System.Drawing.Point(24, 16);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(128, 16);
this.label2.TabIndex = 7;
this.label2.Text = "Host Name";
//
// TestDNS
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(496, 126);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.label2,
this.combIPAddress,
this.label1,
this.hostName,
this.ButtonByName,
this.ButtonByAddress});
this.Name = "TestDNS";
this.Text = "TestDNS";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new TestDNS());
}
private void OnByAddress(object sender, System.Windows.Forms.MouseEventArgs e)
{
Cursor cursor = this.Cursor;
try
{
// カーソルを WaitCursor にする。
this.Cursor = Cursors.WaitCursor;
System.Net.IPHostEntry ipEntry = System.Net.Dns.GetHostByAddress(this.combIPAddress.Text);
this.hostName.Text = ipEntry.HostName;
}
catch (System.Exception exc)
{
// フォーマットエラーなどの例外処理
MessageBox.Show(exc.Message, "TestDNS", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
}
finally
{
// カーソルを元に戻す。
this.Cursor = cursor;
}
}
private void OnByName(object sender, System.Windows.Forms.MouseEventArgs e)
{
Cursor cursor = this.Cursor;
try
{
// カーソルを WaitCursor にする。
this.Cursor = Cursors.WaitCursor;
System.Net.IPHostEntry ipEntry = System.Net.Dns.GetHostByName(this.hostName.Text);
// ちらつきを防止するために描画を停止する。
this.combIPAddress.BeginUpdate();
// リストボックスをクリアする。
this.combIPAddress.Items.Clear();
foreach (IPAddress i in ipEntry.AddressList)
{
this.combIPAddress.Items.Add( i.ToString() );
}
this.combIPAddress.Text = this.combIPAddress.Items[0].ToString();
}
catch (System.Exception exc)
{
// フォーマットエラーなどの例外処理
MessageBox.Show(exc.Message, "TestDNS", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
}
finally
{
// 停止していた描画を再開する。
this.combIPAddress.EndUpdate();
// カーソルを元に戻す。
this.Cursor = cursor;
}
}
}
}