|

XML テキストファイルのリード・ライト
|
開発環境: Visual Studio 2003次のような機能のForm アプリケーションを作る。
- XMLファイルを書き出す。
- 書き出したファイルを読み込み、RichTextBoxに書き出す。
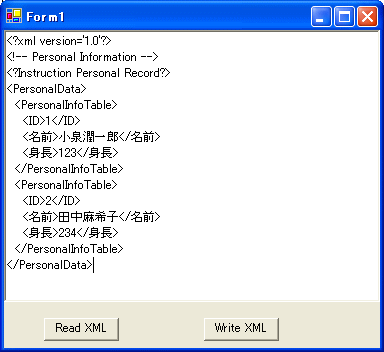
WRITE 時のヒント |
エンコーディングを指定する。 <?xml version="1.0"
encoding="utf-8"?> |
XmlTextWriter xtw = new System.Xml.XmlTextWriter(dialog.FileName
System.Text.Encoding.UTF8); |
インデントをつけて書き出す。 |
xtw.Formatting = Formatting.Indented; |
インデント幅の指定 |
xtw.Indentation = 4; |
日付 | 修正履歴 |
2002/6/22 | 初期バージョン作成 |
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Xml;
namespace XMLReaderWriter
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.RichTextBox richTextBox1;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.richTextBox1 = new System.Windows.Forms.RichTextBox();
this.SuspendLayout();
//
// button1
//
this.button1.Location = new System.Drawing.Point(40, 288);
this.button1.Name = "button1";
this.button1.TabIndex = 0;
this.button1.Text = "Read XML";
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// button2
//
this.button2.Location = new System.Drawing.Point(200, 288);
this.button2.Name = "button2";
this.button2.TabIndex = 1;
this.button2.Text = "Write XML";
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// richTextBox1
//
this.richTextBox1.Dock = System.Windows.Forms.DockStyle.Top;
this.richTextBox1.Name = "richTextBox1";
this.richTextBox1.Size = new System.Drawing.Size(376, 272);
this.richTextBox1.TabIndex = 2;
this.richTextBox1.Text = "";
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(376, 318);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.richTextBox1,
this.button2,
this.button1});
this.Name = "Form1";
this.Text = "Form1";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void button1_Click(object sender, System.EventArgs e)
{
OpenFileDialog dialog = new OpenFileDialog();
DialogResult result = dialog.ShowDialog();
if (result == DialogResult.OK)
{
XmlTextReader xtr = new System.Xml.XmlTextReader(dialog.FileName);
this.richTextBox1.Text = "";
while(xtr.Read())
{
switch (xtr.NodeType)
{
case XmlNodeType.CDATA:
this.richTextBox1.Text += string.Format("<![CDATA[{0}]]>", xtr.Value);
break;
case XmlNodeType.Comment:
this.richTextBox1.Text += string.Format("<!-- {0} -->", xtr.Value);
break;
case XmlNodeType.DocumentType:
this.richTextBox1.Text += string.Format("<!DOCTYPE {0} [{1}]", xtr.Name, xtr.Value);
break;
case XmlNodeType.Element:
this.richTextBox1.Text += "<" + xtr.Name;
while (xtr.MoveToNextAttribute())
this.richTextBox1.Text += " " + xtr.Name + "='" + xtr.Value + "'";
this.richTextBox1.Text += ">";
break;
case XmlNodeType.EndElement:
this.richTextBox1.Text += string.Format("</{0}>", xtr.Name);
break;
case XmlNodeType.EntityReference:
this.richTextBox1.Text += string.Format(xtr.Name);
break;
case XmlNodeType.ProcessingInstruction:
this.richTextBox1.Text += string.Format("<?{0} {1}?>", xtr.Name, xtr.Value);
break;
case XmlNodeType.Whitespace:
this.richTextBox1.Text += xtr.Value;
break;
case XmlNodeType.Text:
this.richTextBox1.Text += string.Format(xtr.Value);
break;
case XmlNodeType.XmlDeclaration:
this.richTextBox1.Text += string.Format("<?xml version='1.0'?>");
break;
default:
this.richTextBox1.Text += string.Format("// Unhandled type : {0}", xtr.NodeType.ToString());
break;
}
}
}
}
private void button2_Click(object sender, System.EventArgs e)
{
SaveFileDialog dialog = new SaveFileDialog();
DialogResult result = dialog.ShowDialog();
if (result == DialogResult.OK)
{
try
{
XmlTextWriter xtw = new System.Xml.XmlTextWriter(dialog.FileName, System.Text.Encoding.UTF8);
xtw.Formatting = Formatting.Indented;
// Starts a new document
xtw.WriteStartDocument();
xtw.WriteComment("Personal Information");
xtw.WriteProcessingInstruction("Instruction","Personal Record");
// PersonalData
xtw.WriteStartElement("PersonalData");
// PersonalInfoTable
xtw.WriteStartElement("PersonalInfoTable");
xtw.WriteElementString("ID", "1");
xtw.WriteElementString("名前", "小泉潤一郎");
xtw.WriteElementString("身長", "123");
xtw.WriteEndElement();
// End of PersonalInfoTable
// PersonalInfoTable
xtw.WriteStartElement("PersonalInfoTable");
xtw.WriteElementString("ID", "2");
xtw.WriteElementString("名前", "田中麻希子");
xtw.WriteElementString("身長", "234");
xtw.WriteEndElement();
// End of PersonalInfoTable
// End of PersonalData
xtw.WriteEndElement();
// EndDocument
xtw.WriteEndDocument();
xtw.Close();
}
catch (Exception exc)
{
MessageBox.Show(exc.Message, // テキスト
exc.GetType().ToString(), // キャプション
MessageBoxButtons.OK,
MessageBoxIcon.Exclamation);
}
}
}
}
}