|
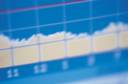
任意のパフォーマンスを表示する
|
開発環境: Visual Studio 2003次のような機能のForm アプリケーションを作る。
- パフォーマンス情報を1秒ごとに プログレスバー に表示する。
- ダイアログにより任意のカウンターを選択できる。
パフォーマンスメータ本体。
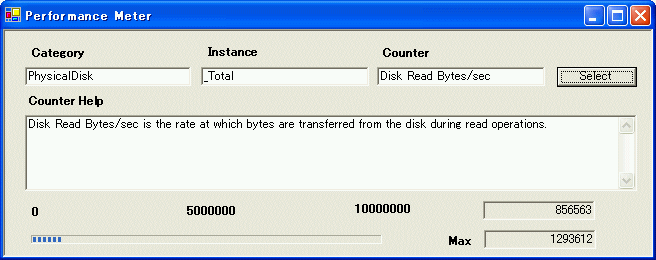
カウンターピッカー
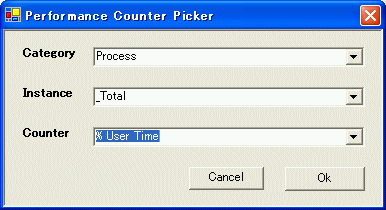
作り方
0. まずは、パフォーマンスカウンターに、Category, Instance, Counter という階層構造があるので、それを理解する必要がある。
PerformanceCounter
分類 | 例 |
Category | Process, PhysicalDiskなど最も上位のカテゴリー |
Instance | プロセス名、C:, などドライブ名などのインスタンス |
Counter | % User Time, Avg. Disk Bytes/Read などの最終的カウンタ |
1. static
メソッド System.Diagnostics.PerformanceCounterCategory.GetCategories();
により、PerformanceCounterCategory[] のカテゴリーテーブルを取得する。
2. カテゴリーテーブルより、表示したいカテゴリ(Processなど)を選択する。
3. categoryInstance = new PerformanceCounterCategory(categoryName);
により、カテゴリーのインスタンスを作成する。
4. string instanceNames[] =
categoryInstance.GetInstanceNames();により、インスタンス名の配列を取得する。
5. このインスタンス配列から表示したいものを選ぶ。インスタンスが存在しない場合もあるので注意。
6. PerformanceCounter[] =
categoryInstance.GetCounters(this.instanceName)により、カウンターの配列を取得する。
7. 参照したいカウンターインスタンスに対し、GetNext() により値を取得する。
注意1:
Help を見る限りでは、簡単に書けそうだったのだけれど、はまりました。
原因は、Category を選択すると、それに対応したインスタンステーブルを取得する必要がある。
このため、インスタンステーブルを取得しなおしが発生する。
さらに、インスタンスを選択すると、それに対応したカウンターテーブルを取得する必要がある。
このため、インスタンステーブルを取得しなおしが発生する。
このため、依存関係がきれいに整理できまず、きれいにかけませんでした。
注意2:
カウンターの種類によっては、インスタンスが存在しないものがあり、GetInstanceNames を呼ぶと例外があがる。
注意3;
Form1.cs, Form2.cs に分けて書きましたが、カウンターの受け渡しは
public PerformanceCounter のパブリックで渡しています。(手抜き)
注意4:
とりあえず忘れてしまいそうなので、大事そうなところだけメモっておきました。
プログラム自体も十分なテストをしてないのでバグありです。きっと (^^)/
詳しくは、Help を参照。
PerformanceCounter
分類 | 例 |
Form1.cs | パフォーマンスメータ本体 |
Form2.cs | パフォーマンスカウンターを選択するためのモーダルダイアログ |
Form1.cs
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Diagnostics;
namespace PerfMeter
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.Timer timer1;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Label label5;
private System.Windows.Forms.Label label6;
private System.Windows.Forms.Label label7;
private System.Windows.Forms.Label label8;
private System.Windows.Forms.TextBox tbCategory;
private System.Windows.Forms.TextBox tbInstance;
private System.Windows.Forms.TextBox tbCounterHelp;
private System.Windows.Forms.TextBox tbCounter;
private System.Windows.Forms.ErrorProvider errorProvider1;
private System.Windows.Forms.Label labelMid;
private System.Windows.Forms.Label labelMax;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.ProgressBar progressBar1;
private System.Windows.Forms.TextBox textBoxMax;
private System.ComponentModel.IContainer components;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
this.timer1 = new System.Windows.Forms.Timer(this.components);
this.label3 = new System.Windows.Forms.Label();
this.textBox1 = new System.Windows.Forms.TextBox();
this.button1 = new System.Windows.Forms.Button();
this.tbCategory = new System.Windows.Forms.TextBox();
this.tbInstance = new System.Windows.Forms.TextBox();
this.tbCounterHelp = new System.Windows.Forms.TextBox();
this.tbCounter = new System.Windows.Forms.TextBox();
this.labelMid = new System.Windows.Forms.Label();
this.labelMax = new System.Windows.Forms.Label();
this.label5 = new System.Windows.Forms.Label();
this.label6 = new System.Windows.Forms.Label();
this.label7 = new System.Windows.Forms.Label();
this.label8 = new System.Windows.Forms.Label();
this.errorProvider1 = new System.Windows.Forms.ErrorProvider();
this.textBoxMax = new System.Windows.Forms.TextBox();
this.label1 = new System.Windows.Forms.Label();
this.progressBar1 = new System.Windows.Forms.ProgressBar();
this.SuspendLayout();
//
// timer1
//
this.timer1.Interval = 1000;
this.timer1.Tick += new System.EventHandler(this.OnTick);
//
// label3
//
this.label3.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label3.Location = new System.Drawing.Point(25, 175);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(14, 14);
this.label3.TabIndex = 6;
this.label3.Text = "0";
//
// textBox1
//
this.textBox1.Anchor = (System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Left);
this.textBox1.Location = new System.Drawing.Point(478, 170);
this.textBox1.Name = "textBox1";
this.textBox1.ReadOnly = true;
this.textBox1.Size = new System.Drawing.Size(112, 19);
this.textBox1.TabIndex = 7;
this.textBox1.Text = "";
this.textBox1.TextAlign = System.Windows.Forms.HorizontalAlignment.Right;
//
// button1
//
this.button1.Location = new System.Drawing.Point(552, 36);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(80, 20);
this.button1.TabIndex = 8;
this.button1.Text = "Select";
this.button1.Click += new System.EventHandler(this.OnButton1);
//
// tbCategory
//
this.tbCategory.Location = new System.Drawing.Point(20, 36);
this.tbCategory.Name = "tbCategory";
this.tbCategory.Size = new System.Drawing.Size(166, 19);
this.tbCategory.TabIndex = 9;
this.tbCategory.Text = "";
//
// tbInstance
//
this.tbInstance.Location = new System.Drawing.Point(196, 36);
this.tbInstance.Name = "tbInstance";
this.tbInstance.Size = new System.Drawing.Size(168, 19);
this.tbInstance.TabIndex = 10;
this.tbInstance.Text = "";
//
// tbCounterHelp
//
this.tbCounterHelp.Location = new System.Drawing.Point(20, 84);
this.tbCounterHelp.Multiline = true;
this.tbCounterHelp.Name = "tbCounterHelp";
this.tbCounterHelp.ScrollBars = System.Windows.Forms.ScrollBars.Vertical;
this.tbCounterHelp.Size = new System.Drawing.Size(612, 76);
this.tbCounterHelp.TabIndex = 11;
this.tbCounterHelp.Text = "";
//
// tbCounter
//
this.tbCounter.Location = new System.Drawing.Point(372, 36);
this.tbCounter.Name = "tbCounter";
this.tbCounter.Size = new System.Drawing.Size(168, 19);
this.tbCounter.TabIndex = 12;
this.tbCounter.Text = "";
//
// labelMid
//
this.labelMid.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.labelMid.Location = new System.Drawing.Point(162, 174);
this.labelMid.Name = "labelMid";
this.labelMid.Size = new System.Drawing.Size(84, 14);
this.labelMid.TabIndex = 14;
this.labelMid.Text = "50";
this.labelMid.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// labelMax
//
this.labelMax.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.labelMax.Location = new System.Drawing.Point(325, 172);
this.labelMax.Name = "labelMax";
this.labelMax.Size = new System.Drawing.Size(102, 14);
this.labelMax.TabIndex = 15;
this.labelMax.Text = "100";
this.labelMax.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label5
//
this.label5.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label5.Location = new System.Drawing.Point(25, 16);
this.label5.Name = "label5";
this.label5.Size = new System.Drawing.Size(60, 14);
this.label5.TabIndex = 17;
this.label5.Text = "Category";
//
// label6
//
this.label6.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label6.Location = new System.Drawing.Point(201, 15);
this.label6.Name = "label6";
this.label6.Size = new System.Drawing.Size(60, 14);
this.label6.TabIndex = 18;
this.label6.Text = "Instance";
//
// label7
//
this.label7.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label7.Location = new System.Drawing.Point(376, 16);
this.label7.Name = "label7";
this.label7.Size = new System.Drawing.Size(60, 14);
this.label7.TabIndex = 19;
this.label7.Text = "Counter";
//
// label8
//
this.label8.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label8.Location = new System.Drawing.Point(22, 64);
this.label8.Name = "label8";
this.label8.Size = new System.Drawing.Size(85, 14);
this.label8.TabIndex = 20;
this.label8.Text = "Counter Help";
//
// errorProvider1
//
this.errorProvider1.DataMember = null;
//
// textBoxMax
//
this.textBoxMax.Anchor = (System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Left);
this.textBoxMax.Location = new System.Drawing.Point(479, 199);
this.textBoxMax.Name = "textBoxMax";
this.textBoxMax.ReadOnly = true;
this.textBoxMax.Size = new System.Drawing.Size(112, 19);
this.textBoxMax.TabIndex = 22;
this.textBoxMax.Text = "";
this.textBoxMax.TextAlign = System.Windows.Forms.HorizontalAlignment.Right;
//
// label1
//
this.label1.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label1.Location = new System.Drawing.Point(440, 204);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(27, 14);
this.label1.TabIndex = 23;
this.label1.Text = "Max";
this.label1.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// progressBar1
//
this.progressBar1.Location = new System.Drawing.Point(26, 204);
this.progressBar1.Name = "progressBar1";
this.progressBar1.Size = new System.Drawing.Size(351, 9);
this.progressBar1.TabIndex = 24;
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(646, 224);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.progressBar1,
this.label1,
this.textBoxMax,
this.label8,
this.label7,
this.label6,
this.label5,
this.labelMax,
this.labelMid,
this.tbCounter,
this.tbCounterHelp,
this.tbInstance,
this.tbCategory,
this.button1,
this.textBox1,
this.label3});
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.Fixed3D;
this.Name = "Form1";
this.Text = "Performance Meter";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private PerformanceCounter performanceCounter1 = null;
private int MaxValue = 0;
private void OnTick(object sender, System.EventArgs e)
{
try
{
int val = (int)this.performanceCounter1.NextValue();
while (val > 0)
{
if ( val >= this.progressBar1.Maximum)
this.progressBar1.Maximum *= 10;
else
break;
}
if (val > this.MaxValue)
{
this.MaxValue = val;
this.textBoxMax.Text = val.ToString();
}
this.labelMid.Text = (this.progressBar1.Maximum/2).ToString();
this.labelMax.Text = (this.progressBar1.Maximum).ToString();
this.progressBar1.Value = val;
this.textBox1.Text = val.ToString();
}
catch (System.ArgumentException exp)
{
this.errorProvider1.SetError(this.progressBar1, "Out of Range!" + exp.Message);
}
}
private void OnButton1(object sender, System.EventArgs e)
{
this.timer1.Enabled = false;
Form2 form = new Form2();
form.ShowDialog();
this.performanceCounter1 = form.perfC;
this.tbCategory.Text = this.performanceCounter1.CategoryName;
this.tbInstance.Text = this.performanceCounter1.InstanceName;
this.tbCounter.Text = this.performanceCounter1.CounterName;
this.tbCounterHelp.Text = this.performanceCounter1.CounterHelp;
this.timer1.Enabled = true;
this.MaxValue = 0;
}
}
}
Form2.cs
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Diagnostics;
namespace PerfMeter
{
/// <summary>
/// Form2 の概要の説明です。
/// </summary>
public class Form2 : System.Windows.Forms.Form
{
private System.Windows.Forms.Label label5;
private System.Windows.Forms.Label label4;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.ComboBox comboBoxCounters;
private System.Windows.Forms.ComboBox comboBoxInstances;
private System.Windows.Forms.ComboBox comboBoxCategories;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
/// <summary>
/// 必要なデザイナ変数です。
/// </summary>
private System.ComponentModel.Container components = null;
public Form2()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
this.InitCategoryTable();
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.label5 = new System.Windows.Forms.Label();
this.label4 = new System.Windows.Forms.Label();
this.label1 = new System.Windows.Forms.Label();
this.comboBoxCounters = new System.Windows.Forms.ComboBox();
this.comboBoxInstances = new System.Windows.Forms.ComboBox();
this.comboBoxCategories = new System.Windows.Forms.ComboBox();
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// label5
//
this.label5.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label5.Location = new System.Drawing.Point(16, 96);
this.label5.Name = "label5";
this.label5.Size = new System.Drawing.Size(56, 16);
this.label5.TabIndex = 19;
this.label5.Text = "Counter";
//
// label4
//
this.label4.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label4.Location = new System.Drawing.Point(16, 56);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(72, 16);
this.label4.TabIndex = 18;
this.label4.Text = "Instance";
//
// label1
//
this.label1.Font = new System.Drawing.Font("MS UI Gothic", 9F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(128)));
this.label1.Location = new System.Drawing.Point(16, 16);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(56, 16);
this.label1.TabIndex = 17;
this.label1.Text = "Category";
//
// comboBoxCounters
//
this.comboBoxCounters.Enabled = false;
this.comboBoxCounters.Location = new System.Drawing.Point(88, 96);
this.comboBoxCounters.Name = "comboBoxCounters";
this.comboBoxCounters.Size = new System.Drawing.Size(272, 20);
this.comboBoxCounters.Sorted = true;
this.comboBoxCounters.TabIndex = 16;
this.comboBoxCounters.SelectedIndexChanged += new System.EventHandler(this.OnCounterChanged);
//
// comboBoxInstances
//
this.comboBoxInstances.Enabled = false;
this.comboBoxInstances.Location = new System.Drawing.Point(88, 56);
this.comboBoxInstances.Name = "comboBoxInstances";
this.comboBoxInstances.Size = new System.Drawing.Size(272, 20);
this.comboBoxInstances.Sorted = true;
this.comboBoxInstances.TabIndex = 15;
this.comboBoxInstances.SelectedIndexChanged += new System.EventHandler(this.OnInstanceChanged);
//
// comboBoxCategories
//
this.comboBoxCategories.Location = new System.Drawing.Point(88, 16);
this.comboBoxCategories.Name = "comboBoxCategories";
this.comboBoxCategories.Size = new System.Drawing.Size(272, 20);
this.comboBoxCategories.Sorted = true;
this.comboBoxCategories.TabIndex = 14;
this.comboBoxCategories.SelectedIndexChanged += new System.EventHandler(this.OnCategoryChanged);
//
// button1
//
this.button1.Location = new System.Drawing.Point(280, 136);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(80, 24);
this.button1.TabIndex = 20;
this.button1.Text = "Ok";
this.button1.Click += new System.EventHandler(this.OnOK);
//
// button2
//
this.button2.Location = new System.Drawing.Point(184, 136);
this.button2.Name = "button2";
this.button2.TabIndex = 21;
this.button2.Text = "Cancel";
this.button2.Click += new System.EventHandler(this.OnCancel);
//
// Form2
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(376, 174);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.button2,
this.button1,
this.label5,
this.label4,
this.label1,
this.comboBoxCounters,
this.comboBoxInstances,
this.comboBoxCategories});
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.Fixed3D;
this.MaximizeBox = false;
this.MinimizeBox = false;
this.Name = "Form2";
this.Text = "Performance Counter Picker";
this.ResumeLayout(false);
}
#endregion
private PerformanceCounterCategory[] categoryTable = null;
private string categoryName = "Process";
private string instanceName = "_Total";
private string counterName = "% User Time";
private System.Diagnostics.PerformanceCounterCategory perfCat = null;
private PerformanceCounter[] perfCounters = null;
public PerformanceCounter perfC;
private void InitCategoryTable()
{
categoryTable = System.Diagnostics.PerformanceCounterCategory.GetCategories();
foreach(PerformanceCounterCategory cat in categoryTable)
{
this.comboBoxCategories.Items.Add(cat.CategoryName);
}
this.comboBoxCategories.SelectedIndex
= this.comboBoxCategories.Items.IndexOf(categoryName);
this.InitInstanceTable();
}
private void InitInstanceTable()
{
perfCat = new PerformanceCounterCategory(this.categoryName);
string[] instanceNames = null;
try
{
instanceNames = perfCat.GetInstanceNames();
}
catch (Exception)
{
this.comboBoxInstances.Enabled = false;
this.comboBoxCounters.Enabled = false;
return;
}
this.comboBoxInstances.Items.Clear();
if(instanceNames.Length == 0)
{
this.comboBoxInstances.Enabled = false;
}
else
{
this.comboBoxInstances.Enabled = true;
foreach(string instanceName in instanceNames)
{
this.comboBoxInstances.Items.Add(instanceName);
}
}
}
private void InitCounterTable()
{
this.comboBoxCounters.Items.Clear();
try
{
perfCounters =
perfCat.GetCounters(this.instanceName);
}
catch(Exception)
{
this.comboBoxCounters.Enabled = false;
return;
}
foreach(PerformanceCounter perfCounter in perfCounters)
{
this.comboBoxCounters.Items.Add(perfCounter.CounterName);
}
this.comboBoxCounters.Enabled = true;
}
private void OnCategoryChanged(object sender, System.EventArgs e)
{
this.categoryName =
this.comboBoxCategories.Text;
this.InitInstanceTable();
}
private void OnInstanceChanged(object sender, System.EventArgs e)
{
this.instanceName =
this.comboBoxInstances.Text;
this.InitCounterTable();
}
private void OnCounterChanged(object sender, System.EventArgs e)
{
this.counterName = this.comboBoxCounters.Text;
}
private void OnOK(object sender, System.EventArgs e)
{
foreach(PerformanceCounter perfCounter in perfCounters)
{
if (perfCounter.CounterName == this.counterName)
{
this.perfC = perfCounter;
this.Close();
}
}
}
private void OnCancel(object sender, System.EventArgs e)
{
this.Close();
}
}
}