目次 |
- 目的
- 参考URL
- 作り方
- ソースコード
- その他の作り方1
- その他の作り方2
|
|
自分で作成したWindows.Forms アプリケーションのカスタムパフォーマンスカウンターを作成します。
これにより、次のような パフォーマンスメータ (スタート→すべてのプログラム→管理ツール→パフォーマンス)で、自分のパフォーマンスカウンターをモニターできるようになります。
パフォーマンスメータを利用できるようになるので、チューニングが必要なアプリケーションではとても便利に使えそうです。
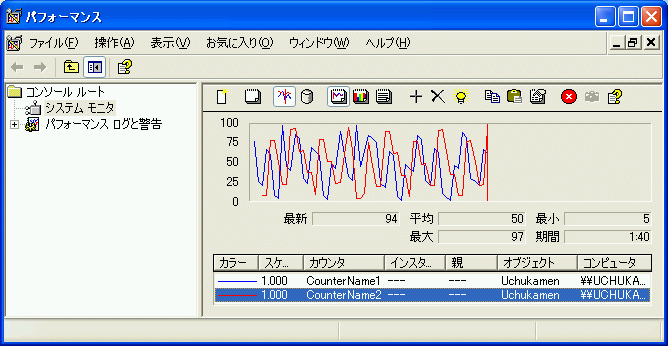
|
|
(1) カスタム
パフォーマンス カウンタの作成 (VS.NET のヘルプ)
(2) パフォーマンス
カウンタのカテゴリの作成 (VS.NET のヘルプ)
(3) パフォーマンス
カウンタの型 (VS.NET のヘルプ)
|
|
1, Windows.Forms アプリケーションを新規作成します。
2. サーバエクスプローラで、サーバ→コンピュータ名→パフォーマンスカウンタを右クリックし、新しいカテゴリの作成を選択します。
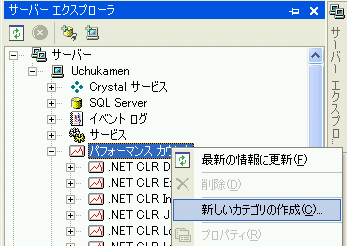
3. すると、つぎのようなパフォーマンスカウンタビルダが表示されます。
そこで、次のようにカテゴリ名、カテゴリの説明を入力します。
さらに、新規作成ボタンを押して、カウンタを追加します。
ここでは、2つのカウンタを作っていますが、1つでももちろんOK。
Note: カテゴリ名に日本語も使えました。
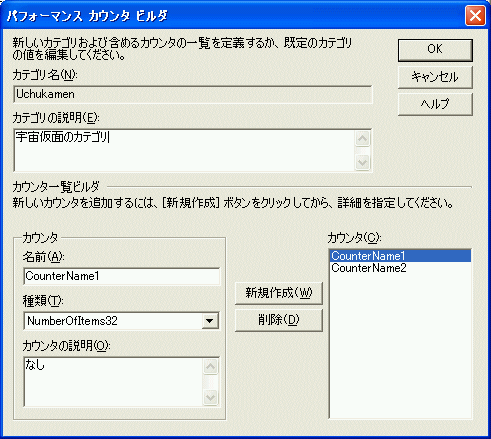
注意: 種類(T)の NumberOfItems32 は、データタイプの指定です。詳細は、パフォーマンス
カウンタの型 (VS.NET のヘルプ)を見て下さい。
4. すると、つぎのようにサーバエクスプローラのパフォーマンスカウンタのところに、Uchukamen
というカテゴリと、CounterName1, CounterName2 というカウンタが生成されます。
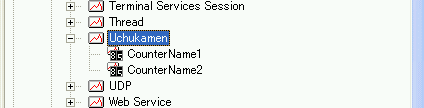
5. この CounterName1, CounterName2 を Windows.Forms にドラッグ&ドロップします。
6. すると、次のように CounterName1, CounterName2 がフォームデザイナー上に現れます。
注意: CounterName1, CounterName2 のプロパティで、ReadOnly
プロパティを False にしないと、カウンターに値をセットできません。
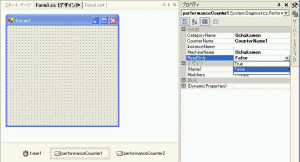
6. あとは、適当なところで、this.performanceCounter1.RawValue = 適当な数値とすればOKです。
ここでは、タイマーで適当な乱数をカウンターにセットするようにします。
タイマーのイベントハンドラーで次のようなコードを書きます。
注意: タイマーの Enabled プロパティを True
にしないと、タイマーが動かない。←つい忘れる。
コードを書く必要があるのは、この3行だけです。
private void timer1_Tick(object sender, System.EventArgs e)
{
System.Random rnd = new System.Random();
this.performanceCounter1.RawValue = rnd.Next(100);
this.performanceCounter2.RawValue = rnd.Next(100);
}
7 コンパイル&RUN !
8. パフォーマンスメータを起動して、カウンタの追加ボタン(+マーク)を押して、パフォーマンスオブジェクトのコンボボックスにUchukamen
が現れるはずです。あとは、CounterName1, CounterName2 を追加すればOK!
注意: カテゴリー、カウンタ名を修正&リコンパイルした場合、パフォーマンスメータの再起動をしないと正しく表示されません。。
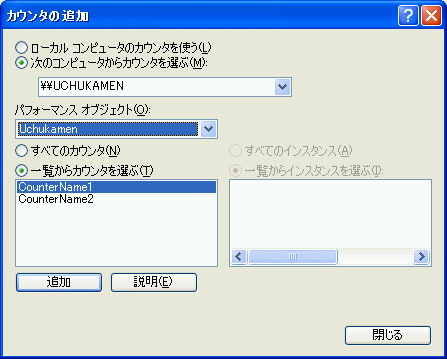
|
|
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace MyPerformanceCounter
{
/// <summary>
/// Form1 の概要の説明です。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.Timer timer1;
private System.Diagnostics.PerformanceCounter performanceCounter1;
private System.Diagnostics.PerformanceCounter performanceCounter2;
private System.ComponentModel.IContainer components;
public Form1()
{
//
// Windows フォーム デザイナ サポートに必要です。
//
InitializeComponent();
//
// TODO: InitializeComponent 呼び出しの後に、コンストラクタ コードを追加してください。
//
}
/// <summary>
/// 使用されているリソースに後処理を実行します。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// デザイナ サポートに必要なメソッドです。このメソッドの内容を
/// コード エディタで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
this.timer1 = new System.Windows.Forms.Timer(this.components);
this.performanceCounter1 = new System.Diagnostics.PerformanceCounter();
this.performanceCounter2 = new System.Diagnostics.PerformanceCounter();
((System.ComponentModel.ISupportInitialize)(this.performanceCounter1)).BeginInit();
((System.ComponentModel.ISupportInitialize)(this.performanceCounter2)).BeginInit();
//
// timer1
//
this.timer1.Enabled = true;
this.timer1.Tick += new System.EventHandler(this.timer1_Tick);
//
// performanceCounter1
//
this.performanceCounter1.CategoryName = "Uchukamen";
this.performanceCounter1.CounterName = "CounterName1";
this.performanceCounter1.MachineName = "Uchukamen";
this.performanceCounter1.ReadOnly = false;
//
// performanceCounter2
//
this.performanceCounter2.CategoryName = "Uchukamen";
this.performanceCounter2.CounterName = "CounterName2";
this.performanceCounter2.MachineName = "Uchukamen";
this.performanceCounter2.ReadOnly = false;
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 12);
this.ClientSize = new System.Drawing.Size(292, 266);
this.Name = "Form1";
this.Text = "Form1";
((System.ComponentModel.ISupportInitialize)(this.performanceCounter1)).EndInit();
((System.ComponentModel.ISupportInitialize)(this.performanceCounter2)).EndInit();
}
#endregion
/// <summary>
/// アプリケーションのメイン エントリ ポイントです。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void timer1_Tick(object sender, System.EventArgs e)
{
System.Random rnd = new System.Random();
this.performanceCounter1.RawValue = rnd.Next(100);
this.performanceCounter2.RawValue = rnd.Next(100);
}
}
}
|
|
CounterCreationData、CounterCreationDataCollection を使ってもできます。
でも、サーバエクスプローラを使うほうが、ラクチンです。
この作り方は、サンプルコードが参考URL(1)に載っているので省略します。
|
|
PerformanceCounterCategory を使ってもできることを確認しました。
でも、カテゴリがすでに登録されているかどうかをチェックしないといけないなど、ちょっと面倒です。
サーバエクスプローラを使うほうが、ラクチンです。
これもパフォーマンス
カウンタのカテゴリの作成に説明が載っているので省略します。
|