1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
// // GameScene.swift import SpriteKit import GameplayKit class GameScene: SKScene { let redBallCategory:UInt32 = 0x001 let blueBallCategory:UInt32 = 0x001 let groundCategory:UInt32 = 0x001 override func didMove(to view: SKView) { let ballRadius: CGFloat = 20 let redBall = SKShapeNode(circleOfRadius: ballRadius) redBall.fillColor = .red redBall.position = CGPoint(x: 280, y: 320) redBall.physicsBody = SKPhysicsBody(circleOfRadius: ballRadius) let blueBall = SKShapeNode(circleOfRadius: ballRadius) blueBall.fillColor = .blue blueBall.position = CGPoint(x: 360, y: 320) blueBall.physicsBody = SKPhysicsBody(circleOfRadius: ballRadius) var splinePoints = [CGPoint(x: 0, y: 300), CGPoint(x: 100, y: 50), CGPoint(x: 400, y: 110), CGPoint(x: 640, y: 20)] let ground = SKShapeNode(splinePoints: &splinePoints, count: splinePoints.count) ground.physicsBody = SKPhysicsBody(edgeChainFrom: ground.path!) ground.physicsBody?.restitution = 0.75 redBall.physicsBody?.collisionBitMask = 0b0001 blueBall.physicsBody?.collisionBitMask = 0b0001 ground.physicsBody?.categoryBitMask = 0b0001 self.addChild(redBall) self.addChild(blueBall) self.addChild(ground) ground.physicsBody?.categoryBitMask = groundCategory redBall.physicsBody?.categoryBitMask = redBallCategory blueBall.physicsBody?.categoryBitMask = blueBallCategory } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
// GameViewController.swift import UIKit import SpriteKit import GameplayKit class GameViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() if let view = self.view as! SKView? { // Load the SKScene from 'GameScene.sks' if let scene = SKScene(fileNamed: "GameScene") { // Present the scene view.presentScene(scene) } view.ignoresSiblingOrder = true view.showsFPS = true view.showsNodeCount = true } } override var shouldAutorotate: Bool { return true } override var supportedInterfaceOrientations: UIInterfaceOrientationMask { if UIDevice.current.userInterfaceIdiom == .phone { return .allButUpsideDown } else { return .all } } override var prefersStatusBarHidden: Bool { return true } } |
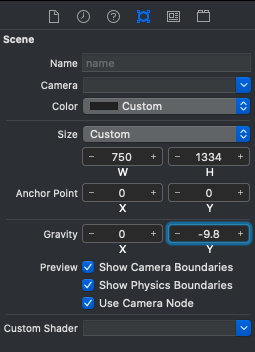
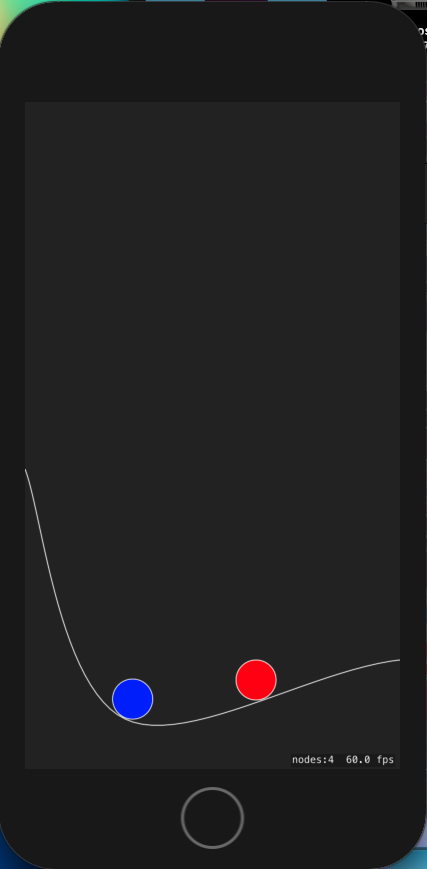